Python Program: Voting eligibility checker
Python Boolean Data Type: Exercise-3 with Solution
Write a program that calculates whether a person is eligible for voting based on their age using boolean conditions.
Sample Solution:
Code:
def voter_eligibility(age):
return age >= 18
def main():
try:
age = int(input("Input your age: "))
if age < 0:
print("Age cannot be negative.")
elif voter_eligibility(age):
print("You are eligible to vote.")
else:
print("You are not eligible to vote.")
except ValueError:
print("Invalid input. Please input a valid age.")
if __name__ == "__main__":
main()
Output:
Input your age: 17 You are not eligible to vote.
Input your age: -14 Age cannot be negative.
Input your age: 18 You are eligible to vote.
When we run the above exercise, it prompts the user to enter their age. Based on the person's age, the "voter_eligibility()" function determines whether they are eligible to vote and prints the appropriate message.
Flowchart:
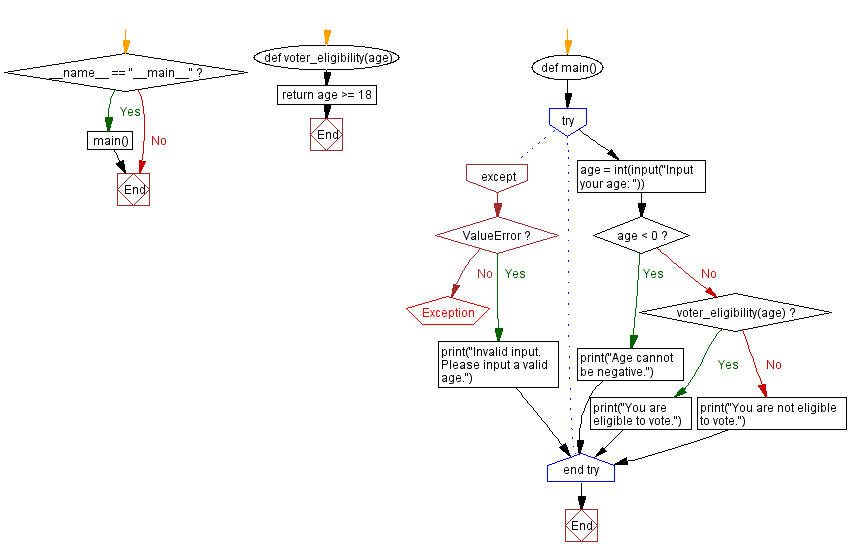
Previous: Python logical AND and OR operations with Boolean values.
Next: Python list empty check using Boolean logics.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics