Python leap year checker using Boolean logic
Python Boolean Data Type: Exercise-5 with Solution
Write a Python program that checks whether a given year is a leap year or not using boolean logic.
Sample Solution:
Code:
def check_leap_year(year):
return (year % 4 == 0 and year % 100 != 0) or (year % 400 == 0)
def main():
try:
year = int(input("Input a valid year: "))
if check_leap_year(year):
print(f"{year} is a leap year.")
else:
print(f"{year} is not a leap year.")
except ValueError:
print("Invalid input. Please input a valid year.")
if __name__ == "__main__":
main()
Output:
Input a valid year: 2000 2000 is a leap year.
Input a valid year: 2021 2021 is not a leap year.
In the above exercise the above program prompts the user to input a year and then uses the "is_leap_year()" function to determine if the year is a leap year or not. It prints the appropriate message based on the results.
Flowchart:
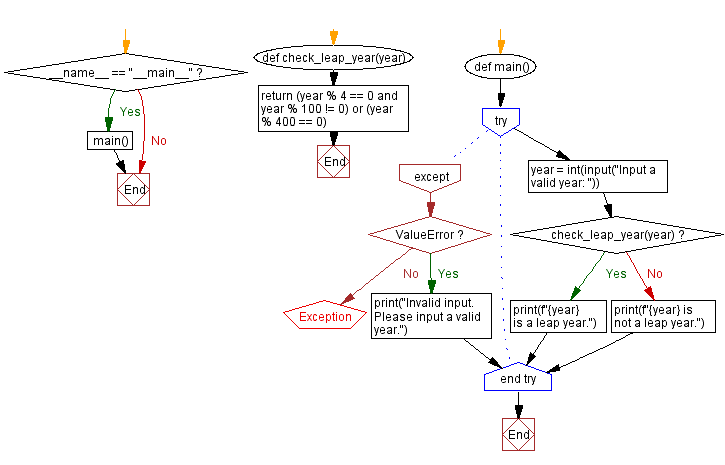
Previous:Python list empty check using Boolean logic.
Next: Python palindrome checker with Boolean logic.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics