Python email validation using Boolean logic
Python Boolean Data Type: Exercise-8 with Solution
Write a Python program that implements a function to check if a given string is a valid email address format using boolean logic.
Sample Solution:
Code:
import re
def is_valid_email(email):
# Regular expression pattern for valid email address format
pattern = r'^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$'
return re.match(pattern, email)
def main():
try:
email = input("Input an email address: ")
if is_valid_email(email):
print("Valid email address!")
else:
print("Invalid email address!")
except Exception as e:
print("An error occurred:", e)
if __name__ == "__main__":
main()
Output:
Input an email address: [email protected] Email address is valid.
Input an email address: [email protected] Email address is valid.
Input an email address: [email protected] Email address is not valid.
In the exercise above the program prompts the user to enter an email address. In order to determine if the email address is valid, it uses the "is_valid_email()" function and a regular expression pattern. It prints the appropriate message based on the results.
Flowchart:
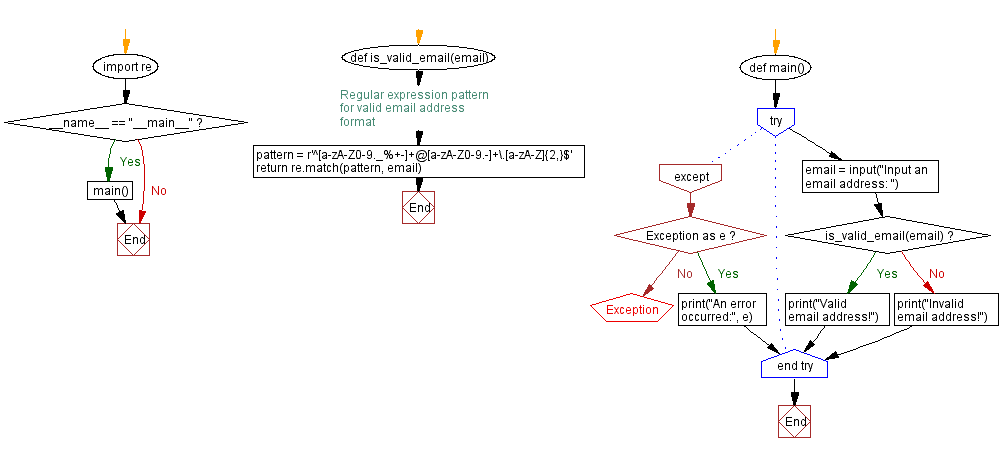
Previous:Python password validation with Boolean expressions.
Next:Python circle area check using Boolean logic.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics