Check disjoint frozensets: Python code and example
Python Frozenset Views Data Type: Exercise-7 with Solution
Write a Python program to check if two frozensets are disjoint (set has no elements in common with other).
Sample Solution:
Code:
def check_disjoint(frozenset_a, frozenset_b):
return frozenset_a.isdisjoint(frozenset_b)
def main():
frozenset_1 = frozenset([1, 2, 3, 4, 5])
frozenset_2 = frozenset([6, 7, 8])
print("Original frozensets:")
print(frozenset_1)
print(frozenset_2)
disjoint_result1 = check_disjoint(frozenset_1, frozenset_2)
if disjoint_result1:
print("The frozensets are disjoint.")
else:
print("The frozensets are not disjoint.")
frozenset_3 = frozenset([1, 2, 3, 4])
frozenset_4 = frozenset([4, 5, 6, 7, 8])
print("\nOriginal frozensets:")
print(frozenset_3)
print(frozenset_4)
disjoint_result2 = check_disjoint(frozenset_3, frozenset_4)
if disjoint_result2:
print("The frozensets are disjoint.")
else:
print("The frozensets are not disjoint.")
if __name__ == "__main__":
main()
Output:
Original frozensets: frozenset({1, 2, 3, 4, 5}) frozenset({8, 6, 7}) The frozensets are disjoint. Original frozensets: frozenset({1, 2, 3, 4}) frozenset({4, 5, 6, 7, 8}) The frozensets are not disjoint.
In this example, the "check_disjoint()" function takes two frozenset instances as input and uses the isdisjoint() method to determine whether they are disjoint or not. The method returns True if the sets have no elements in common, and False otherwise.
The main function creates two sets of frozenset instances and then calls the "check_disjoint()" function to check if they are disjoint. Depending on the result, it prints whether the frozensets are disjoint or not.
Flowchart:
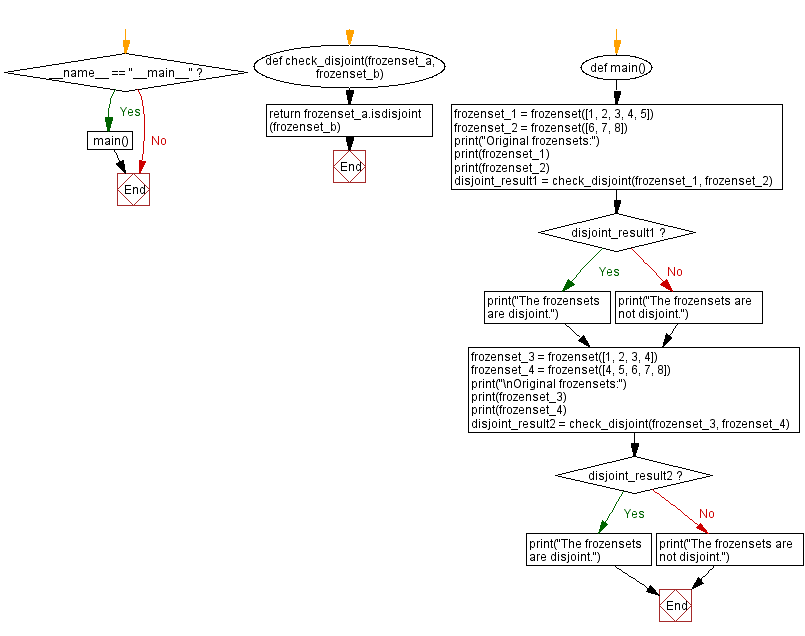
Previous: Create unique frozensets from lists: Python code and example.
Next: Frozenset immutability demonstration: Python code example.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics