Create unique frozensets from lists: Python code and example
6. Frozenset Immutability Demo
Write a Python program that creates a frozenset with unique elements of a list.
Sample Solution:
Code:
def main():
nums = [1, 1, 2, 3, 2, 4, 5, 5, 6, 7, 7]
unique_frozenset_nums = frozenset(nums)
nums1 = [1, 1, 3, 3, 2, 5, 5, -7, -7, 6, 0]
unique_frozenset_nums1 = frozenset(nums1)
print("Original List:", nums)
print("Unique Frozenset:", unique_frozenset_nums)
print("Original List:", nums1)
print("Unique Frozenset:", unique_frozenset_nums1)
if __name__ == "__main__":
main()
Output:
Original List: [1, 1, 2, 3, 2, 4, 5, 5, 6, 7, 7] Unique Frozenset: frozenset({1, 2, 3, 4, 5, 6, 7}) Original List: [1, 1, 3, 3, 2, 5, 5, -7, -7, 6, 0] Unique Frozenset: frozenset({0, 1, 2, 3, 5, 6, -7})
In the exercise above, the 'nums' contains some duplicate elements. The program creates two frozenset named 'unique_frozenset_nums' and 'unique_frozenset_nums1' using the "frozenset()" constructor. The constructor automatically removes duplicate elements from the list, leaving only unique elements.
The program then prints the original list and the resulting unique frozenset. Freezesets are unordered collections of unique elements, so the order of the elements is not guaranteed.
Flowchart:
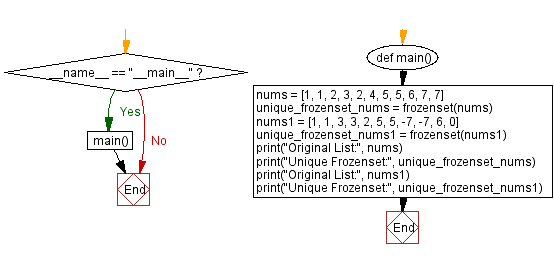
For more Practice: Solve these Related Problems:
- Write a Python program to create a frozenset from a list and attempt to add an element, catching and printing the exception.
- Write a Python script to try removing an element from a frozenset and then print a message confirming its immutability.
- Write a Python program to compare the behavior of a mutable set and a frozenset by attempting modifications on both.
- Write a Python function that accepts a frozenset and prints a custom error message if an attempt is made to modify it.
Go to:
Previous: Python power set generator for frozensets: Code and example.
Next: Check disjoint frozensets: Python code and example.
Python Code Editor :
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.