Python NamedTuple example: Defining a student NamedTuple
Python NamedTuple Data Type: Exercise-4 with Solution
Write a Python program that defines a NamedTuple called Student with fields like name, age, and marks (a list of subjects and marks).
Sample Solution:
Code:
from collections import namedtuple
# Define a NamedTuple named 'Student' with fields 'name', 'age', and 'marks'
Student = namedtuple("Student", ["name", "age", "marks"])
# Create an instance of the Student NamedTuple
student1 = Student("Svetovid Borko", 22, {"Math": 85, "Science": 90, "History": 78, "English": 92, "Art": 88})
student2 = Student("Mohan Balbino", 22, {"Math": 75, "Science": 82, "History": 95, "English": 88, "Art": 90})
student3 = Student("Tacita Jerzy", 21, {"Math": 92, "Science": 87, "History": 85, "English": 78, "Art": 91})
# Access and print fields using dot notation
print("Student 1:")
print("Name:", student1.name)
print("Age:", student1.age)
print("Marks:", student1.marks)
print("\nStudent 2:")
print("Name:", student2.name)
print("Age:", student2.age)
print("Marks:", student2.marks)
print("\nStudent 3:")
print("Name:", student3.name)
print("Age:", student3.age)
print("Marks:", student3.marks)
Output:
Student 1: Name: Svetovid Borko Age: 22 Marks: {'Math': 85, 'Science': 90, 'History': 78, 'English': 92, 'Art': 88} Student 2: Name: Mohan Balbino Age: 22 Marks: {'Math': 75, 'Science': 82, 'History': 95, 'English': 88, 'Art': 90} Student 3: Name: Tacita Jerzy Age: 21 Marks: {'Math': 92, 'Science': 87, 'History': 85, 'English': 78, 'Art': 91}
In the exercise above, we define a NamedTuple named "Student" with the fields 'name', 'age', and 'marks'. The 'marks' field contains a dictionary of subjects and their corresponding marks. Next "Student" NamedTuples are created with different student information, including names, ages, and marks. The fields of each student are then accessed and printed using dot notation.
Flowchart:
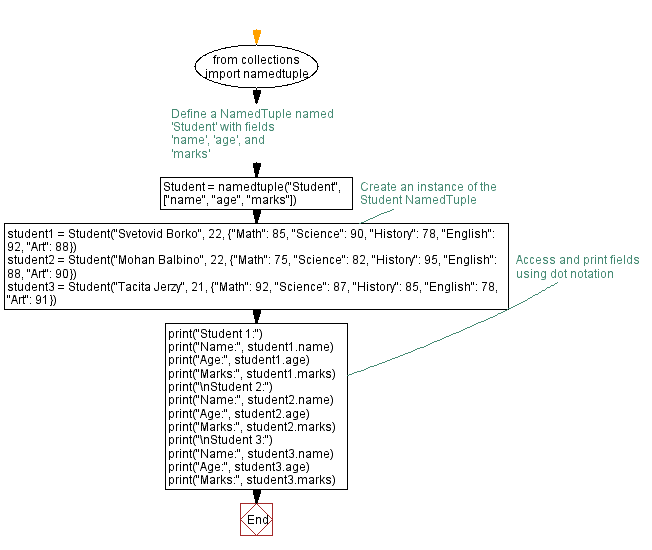
Previous: Python NamedTuple example: Creating a food dictionary.
Next: Python NamedTuple function example: Get item information.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics