Python NamedTuple example: Car attributes
Python NamedTuple Data Type: Exercise-9 with Solution
Write a Python program that defines a NamedTuple named "Car" with fields 'make', 'model', 'year', and 'engine' (a NamedTuple representing engine details). Create an instance of the "Car" NamedTuple and print its attributes.
Sample Solution:
Code:
from collections import namedtuple
# Define a NamedTuple for Engine details
Engine = namedtuple('Engine', ['type', 'cylinders'])
# Define a Car NamedTuple
Car = namedtuple('Car', ['make', 'model', 'year', 'engine'])
# Create an instance of the Engine NamedTuple
engine_instance = Engine(type='1.5L', cylinders=4)
# Create an instance of the Car NamedTuple
car_instance = Car(make='Honda', model='City', year=2020, engine=engine_instance)
# Print the attributes of the Car NamedTuple
print("Car Make:", car_instance.make)
print("Car Model:", car_instance.model)
print("Car Year:", car_instance.year)
print("Car Engine Type:", car_instance.engine.type)
print("Car Engine Cylinders:", car_instance.engine.cylinders)
Output:
Car Make: Honda Car Model: City Car Year: 2020 Car Engine Type: 1.5L Car Engine Cylinders: 4
In the exercise above, we declare a NamedTuple named "Engine" to represent engine details with fields 'type' and 'cylinders'. Then, we define a NamedTuple named "Car" with fields 'make', 'model', 'year', and 'engine'. We create instances of both NamedTuples and use them to create an instance of the "Car" NamedTuple. Lastly, we print the attributes of the "Car" instance, including engine information.
Flowchart:
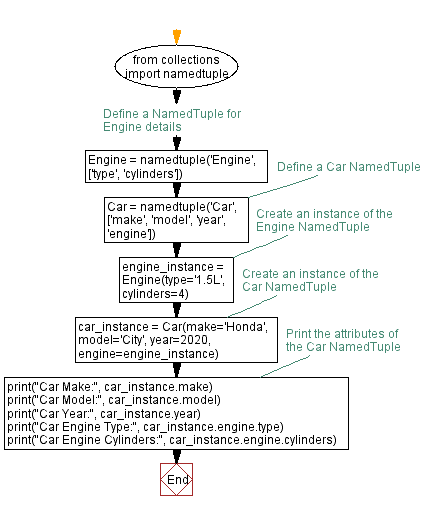
Previous: Python NamedTuple example: Triangle area calculation.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics