Python function: Check descending sort & empty list
Python None Data Type: Exercise-4 with Solution
Write a Python function that checks if a given list is sorted in descending order. Return None if the list is empty.
Sample Solution:
Code:
def is_descending_sorted(input_list):
if not input_list:
return None
sorted_descending = all(input_list[i] >= input_list[i + 1] for i in range(len(input_list) - 1))
return sorted_descending
def main():
try:
# nums = [7, 7, 8, 2, 1]
nums = [1, 2, 3, 4, 5]
result = is_descending_sorted(nums)
if result is None:
print("List is empty.")
elif result:
print("List is sorted in descending order.")
else:
print("List is not sorted in descending order.")
except Exception as e:
print("An error occurred:", e)
if __name__ == "__main__":
main()
Output:
List is not sorted in descending order.
List is not sorted in descending order.
In the above exercise, the "is_descending_sorted()" function checks if the input list is empty. If it's empty, it returns 'None'. Otherwise, it checks if all pairs of adjacent elements are descending by using a generator expression and the "all()" function. If all pairs are in descending order, it returns 'True'; otherwise, it returns 'False'. The "main()" function demonstrates the function's usage by providing a sample list of number.
Flowchart:
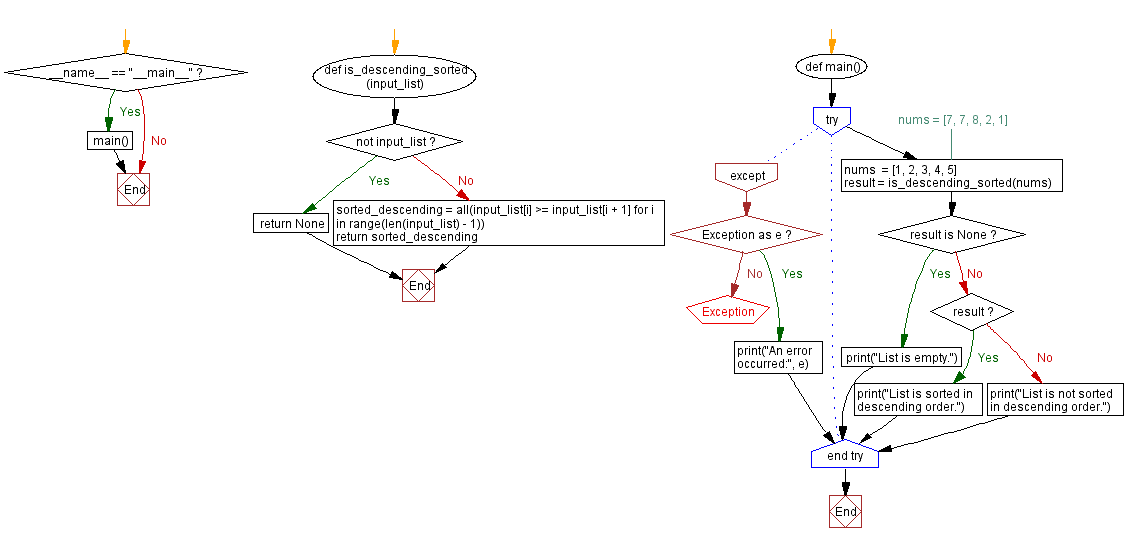
Previous: Python program for handling empty strings.
Next: Handle empty input.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics