Python uppercase converter: Handle empty input
Python None Data Type: Exercise-5 with Solution
Write a Python program that takes a user's input and converts it to uppercase. If the input is empty, return None.
Sample Solution:
Code:
def convert_to_uppercase(input_string):
if not input_string:
return None
return input_string.upper()
def main():
try:
str_input = input("Enter a string: ")
result = convert_to_uppercase(str_input)
if result is None:
print("Input is empty.")
else:
print("Uppercase result:", result)
except Exception as e:
print("An error occurred:", e)
if __name__ == "__main__":
main()
Output:
Enter a string: sdfsd Uppercase result: SDFSD
Enter a string: Input is empty.
In the above exercise, the "convert_to_uppercase()" function checks if the input string is empty. If it's empty, it returns None. Otherwise, it converts the input string to uppercase using the "upper()" method and returns the result. A user input is taken and the result is printed using the "main()" function.
Flowchart:
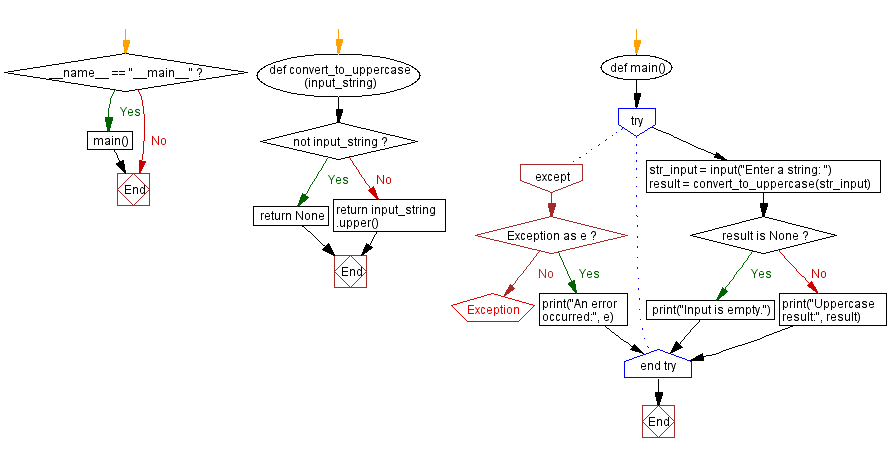
Previous: Check descending sort & empty list.
Next: Python dictionary value retrieval with key check.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics