NumPy: Replace the negative values in a NumPy array with 0
NumPy: Array Object Exercise-90 with Solution
Write a NumPy program to replace the negative values in a NumPy array with 0.
Pictorial Presentation:
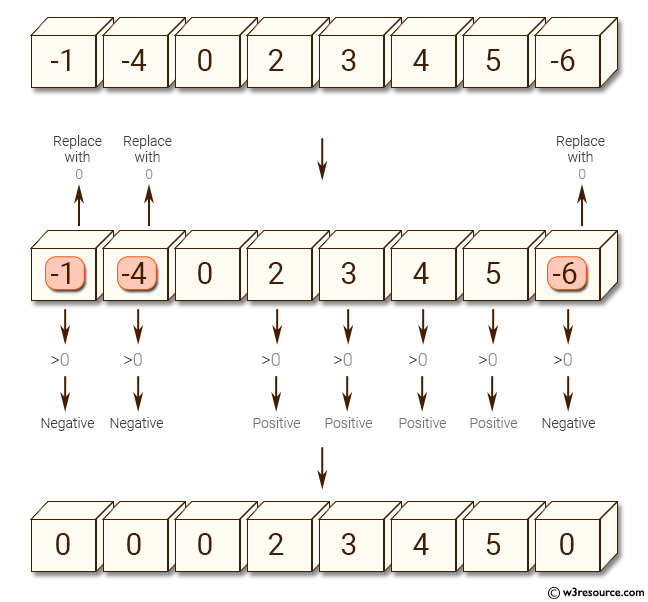
Sample Solution:
Python Code:
# Importing the NumPy library and aliasing it as 'np'
import numpy as np
# Creating a NumPy array 'x' containing integers
x = np.array([-1, -4, 0, 2, 3, 4, 5, -6])
# Printing a message indicating the original array will be displayed
print("Original array:")
# Printing the original array 'x' with its elements
print(x)
# Printing a message indicating the replacement of negative values in the array with 0
print("Replace the negative values of the said array with 0:")
# Replacing all negative values in the array 'x' with 0 using boolean indexing
x[x < 0] = 0
# Printing the modified array 'x' after replacing negative values with 0
print(x)
Sample Output:
Original array: [-1 -4 0 2 3 4 5 -6] Replace the negative values of the said array with 0: [0 0 0 2 3 4 5 0]
Explanation:
In the above code –
x = np.array(...) – This part creates a NumPy array 'x' with the given elements.
x[x < 0] = 0: Use boolean indexing to identify and replace all negative elements in the array 'x' with zeros. The expression x < 0 creates a boolean array with the same shape as 'x', where each element is True if the corresponding element in 'x' is negative and False otherwise. The assignment x[x < 0] = 0 replaces all elements of 'x' for which the corresponding boolean value is True with zeros.
print(x): Print the modified array 'x'.
Python-Numpy Code Editor:
Previous: Write a NumPy program to remove specific elements in a NumPy array.
Next: Write a NumPy program to remove all rows in a NumPy array that contain non-numeric values.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics