NumPy: Remove all rows in a NumPy array that contain non-numeric values
NumPy: Array Object Exercise-91 with Solution
Remove Rows with Non-Numeric Values
Write a NumPy program to remove all rows in a NumPy array that contain non-numeric values.
Pictorial Presentation:
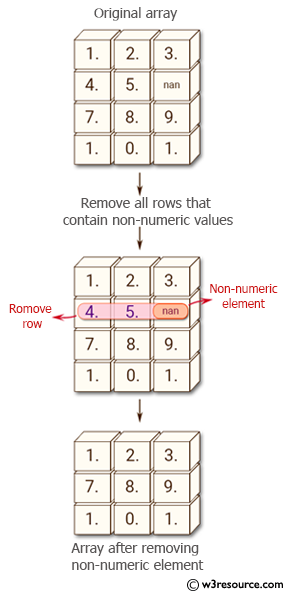
Sample Solution:
Python Code:
# Importing the NumPy library and aliasing it as 'np'
import numpy as np
# Creating a NumPy array 'x' containing various data types including integers, NaN (Not a Number), and booleans
x = np.array([[1, 2, 3], [4, 5, np.nan], [7, 8, 9], [True, False, True]])
# Printing a message indicating the original array will be displayed
print("Original array:")
# Printing the original array 'x' with its elements
print(x)
# Printing a message indicating the removal of all non-numeric elements from the array
print("Remove all non-numeric elements of the said array")
# Removing rows that contain NaN (Not a Number) using np.isnan to check for NaN and 'any' along axis 1 (rows)
# The '~' operator negates the boolean array to select rows where there are no NaN values
result = x[~np.isnan(x).any(axis=1)]
# Printing the resulting array after removing rows with non-numeric elements
print(result)
Sample Output:
Original array: [[ 1. 2. 3.] [ 4. 5. nan] [ 7. 8. 9.] [ 1. 0. 1.]] Remove all non-numeric elements of the said array [[ 1. 2. 3.] [ 7. 8. 9.] [ 1. 0. 1.]]
Explanation:
In the above exercise –
- ‘x = np.array(...)’ creates a 2D NumPy array 'x' with the given elements.
- ‘np.isnan(x)’ creates a boolean array with the same shape as 'x', where each element is True if the corresponding element in 'x' is NaN and False otherwise.
- np.isnan(x).any(axis=1): Use the any() function along axis 1 (rows) to create a 1D boolean array, where each element is True if the corresponding row in 'x' contains at least one NaN value, and False otherwise.
- ~np.isnan(x).any(axis=1): Apply the ~ bitwise negation operator to invert the boolean values in the 1D boolean array. Now, each element is True if the corresponding row in 'x' does not contain any NaN values, and False otherwise.
- x[~np.isnan(x).any(axis=1)]: Use boolean indexing to select only the rows in 'x' for which the corresponding boolean value in the 1D boolean array is True.
- print(...): Finally print() function prints the resulting array, which contains only the rows from 'x' that do not have any NaN values.
Python-Numpy Code Editor:
Previous: Write a NumPy program to replace the negative values in a NumPy array with 0.
Next: Write a NumPy program to select indices satisfying multiple conditions in a NumPy array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/python-exercises/numpy/python-numpy-exercise-91.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics