NumPy: Select indices satisfying multiple conditions in a NumPy array
NumPy: Array Object Exercise-92 with Solution
Write a NumPy program to select indices satisfying multiple conditions in a NumPy array.
Sample array:
a = np.array([97, 101, 105, 111, 117])
b = np.array(['a','e','i','o','u'])
Note: Select the elements from the second array corresponding to elements in the first array that are greater than 100 and less than 110
Pictorial Presentation:
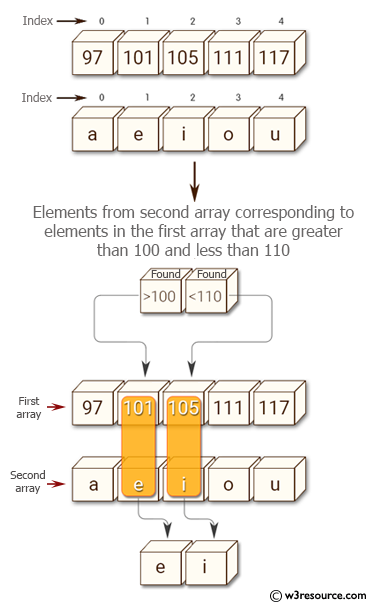
Sample Solution:
Python Code:
# Importing the NumPy library and aliasing it as 'np'
import numpy as np
# Creating a NumPy array 'a' containing integers
a = np.array([97, 101, 105, 111, 117])
# Creating a NumPy array 'b' containing characters representing vowels
b = np.array(['a', 'e', 'i', 'o', 'u'])
# Printing a message indicating the original arrays will be displayed
print("Original arrays")
# Printing the original array 'a' containing integers
print(a)
# Printing the original array 'b' containing characters representing vowels
print(b)
# Printing a message indicating the elements from array 'b' corresponding to specific conditions on array 'a'
print("Elements from the second array corresponding to elements in the first array that are greater than 100 and less than 110:")
# Using boolean indexing to select elements from array 'b' based on conditions from array 'a'
# Selecting elements from array 'b' where the elements in array 'a' are greater than 100 and less than 110
result = b[(100 < a) & (a < 110)]
# Printing the elements from array 'b' corresponding to the specified conditions in array 'a'
print(result)
Sample Output:
Original arrays [ 97 101 105 111 117] ['a' 'e' 'i' 'o' 'u'] Elements from the second array corresponding to elements in the first array that are greater than 100 and less than 110: ['e' 'i']
Explanation:
In the above exercise –
- a = np.array(...): Create a NumPy array 'a' containing integer values.
- b = np.array(...): Create a NumPy array 'b' containing string values. The arrays 'a' and 'b' have the same length.
- (100 < a): This part creates a boolean array with the same shape as 'a', where each element is True if the corresponding element in 'a' is greater than 100, and False otherwise.
- (a < 110): This part creates a boolean array with the same shape as 'a', where each element is True if the corresponding element in 'a' is less than 110, and False otherwise.
- (100 < a) & (a < 110): Use the & operator to perform element-wise logical AND between the two boolean arrays created in steps 3 and 4. The resulting boolean array has True values where the corresponding elements in 'a' are both greater than 100 and less than 110, and False values otherwise.
- b[(100 < a) & (a < 110)]: Use boolean indexing to select elements from array 'b' where the corresponding elements in array 'a' satisfy the condition (greater than 100 and less than 110).
- print(...): Finally print the resulting array.
Python-Numpy Code Editor:
Previous: Write a NumPy program to remove all rows in a NumPy array that contain non-numeric values.
Next: Write a NumPy program to get the magnitude of a vector in NumPy.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics