Python Tkinter CSV viewer with tabular display
Python Tkinter File Operations and Integration: Exercise-2 with Solution
Write a Python program using Tkinter that reads a CSV file and displays its contents in a tabular format, using labels and a grid layout.
Sample Solution:
Python Code:
import tkinter as tk
from tkinter import ttk
import csv
class CSVViewerApp:
def __init__(self, root):
self.root = root
self.root.title("CSV Viewer")
# Create a Frame to hold the table
self.frame = ttk.Frame(root)
self.frame.pack(expand=tk.YES, fill=tk.BOTH)
# Create a Treeview widget for the tabular display
self.tree = ttk.Treeview(self.frame, columns=("Column 1", "Column 2", "Column 3"))
self.tree.heading("#1", text="Column-1")
self.tree.heading("#2", text="Column-2")
self.tree.heading("#3", text="Column-3")
# Add scrollbar for the Treeview
self.scrollbar = ttk.Scrollbar(self.frame, orient="vertical", command=self.tree.yview)
self.tree.configure(yscrollcommand=self.scrollbar.set)
# Pack the Treeview and scrollbar
self.tree.pack(side="left", fill="both", expand=True)
self.scrollbar.pack(side="right", fill="y")
# Open and read the CSV file
with open("test.csv", "r") as csv_file:
csv_reader = csv.reader(csv_file)
# Add data to the Treeview
for row in csv_reader:
self.tree.insert("", "end", values=row)
if __name__ == "__main__":
root = tk.Tk()
app = CSVViewerApp(root)
root.mainloop()
Explanation:
In the exercise above -
The program creates a GUI application using Tkinter with a Treeview widget to display CSV file contents. It reads the CSV file ("test.csv" in this case) and populates the Treeview with its data in a tabular format, where each row of the CSV file corresponds to a row in the table. The columns are labeled "Column 1," "Column 2," and "Column 3," but we can adjust these labels according to our CSV file's structure.
Output:
Flowchart:
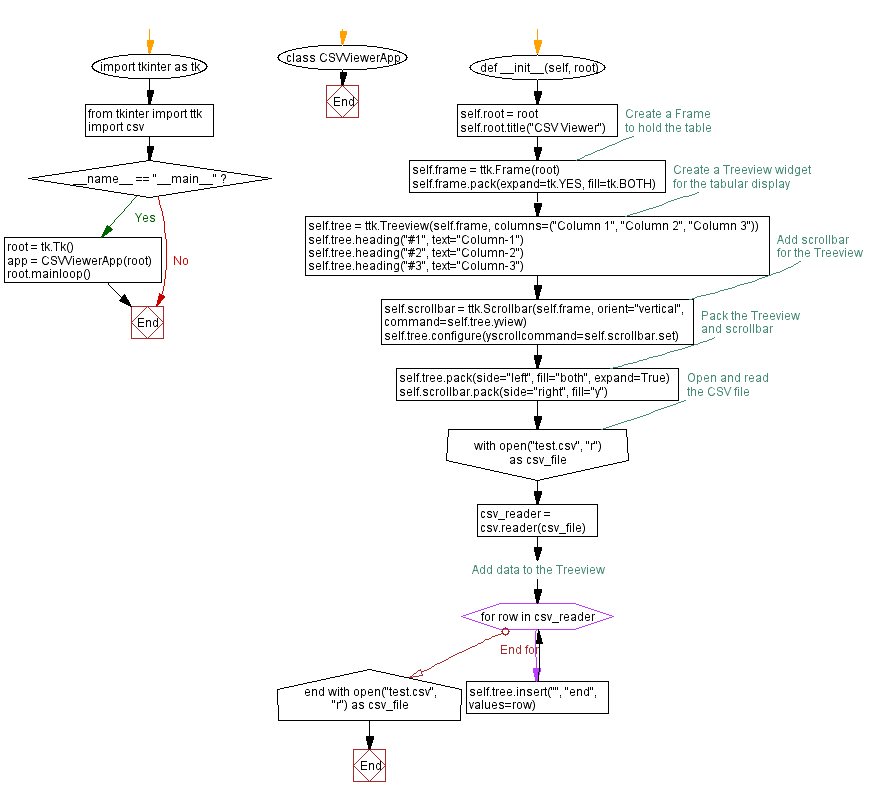
Python Code Editor:
Previous: Python Tkinter text editor app with file operations.
Next: Python Tkinter CSV data entry and writer.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics