Python Tkinter text editor app with file operations
Python Tkinter File Operations and Integration: Exercise-1 with Solution
Write a Python Tkinter application with a text widget that lets users edit and save text files. Include options to create, open, and save files.
Sample Solution:
Python Code:
import tkinter as tk
from tkinter import filedialog
class TextEditorApp:
def __init__(self, root):
self.root = root
self.root.title("Text Editor")
# Create a Text widget
self.text_widget = tk.Text(root)
self.text_widget.pack(fill=tk.BOTH, expand=True)
# Create a Menu bar
menubar = tk.Menu(root)
root.config(menu=menubar)
# File Menu
file_menu = tk.Menu(menubar, tearoff=0)
menubar.add_cascade(label="File", menu=file_menu)
file_menu.add_command(label="New", command=self.new_file)
file_menu.add_command(label="Open", command=self.open_file)
file_menu.add_command(label="Save", command=self.save_file)
file_menu.add_separator()
file_menu.add_command(label="Exit", command=root.destroy)
# Initialize file path
self.file_path = None
def new_file(self):
# Clear the Text widget
self.text_widget.delete(1.0, tk.END)
# Reset the file path
self.file_path = None
def open_file(self):
file_path = filedialog.askopenfilename(filetypes=[("Text Files", "*.txt"), ("All Files", "*.*")])
if file_path:
with open(file_path, "r") as file:
content = file.read()
self.text_widget.delete(1.0, tk.END)
self.text_widget.insert(tk.END, content)
self.file_path = file_path
def save_file(self):
if self.file_path:
content = self.text_widget.get(1.0, tk.END)
with open(self.file_path, "w") as file:
file.write(content)
else:
self.save_file_as()
def save_file_as(self):
file_path = filedialog.asksaveasfilename(defaultextension=".txt", filetypes=[("Text Files", "*.txt"), ("All Files", "*.*")])
if file_path:
content = self.text_widget.get(1.0, tk.END)
with open(file_path, "w") as file:
file.write(content)
self.file_path = file_path
if __name__ == "__main__":
root = tk.Tk()
app = TextEditorApp(root)
root.mainloop()
Explanation:
In the exercise above -
- Import the "tkinter" library to create a graphical user interface (GUI).
- Create a class "TextEditorApp" to represent our text editor application.
- In the init method, initialize the main application window (root) and set its title to "Text Editor."
- Create a 'Text' widget called 'text_widget', which is a multi-line text area where users can edit and view text.
- Create a menu bar (menubar) to hold our file-related operations.
- In the "File" menu, we create options for "New," "Open," "Save," and "Exit." These options are linked to methods that perform the corresponding actions.
- Initialize a variable 'self.file_path' to keep track of the currently opened or saved file's path. It starts as 'None' to indicate that there's no file associated with the editor yet.
- The "new_file()" method clears the 'Text' widget and resets the 'self.file_path' to None.
- The "open_file()" method opens a file dialog, allowing the user to select and open an existing text file. The file's content is read and displayed in the 'Text' widget.
- The "save_file()" method saves the current content in the 'Text' widget to the file specified by 'self.file_path'. If 'self.file_path' is None (i.e., a new file), it calls the "save_file_as()" method.
- The "save_file_as()" method opens a file dialog, allowing the user to specify a file path to save the content. It then writes the content to the chosen file and updates 'self.file_path'.
- In the if name == "__main__": block, start the Tkinter main loop with "root.mainloop()".
Output:
Flowchart:
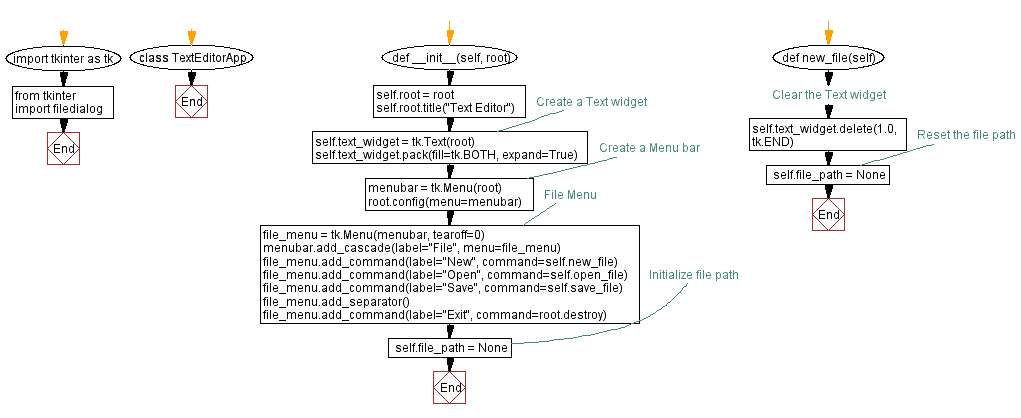
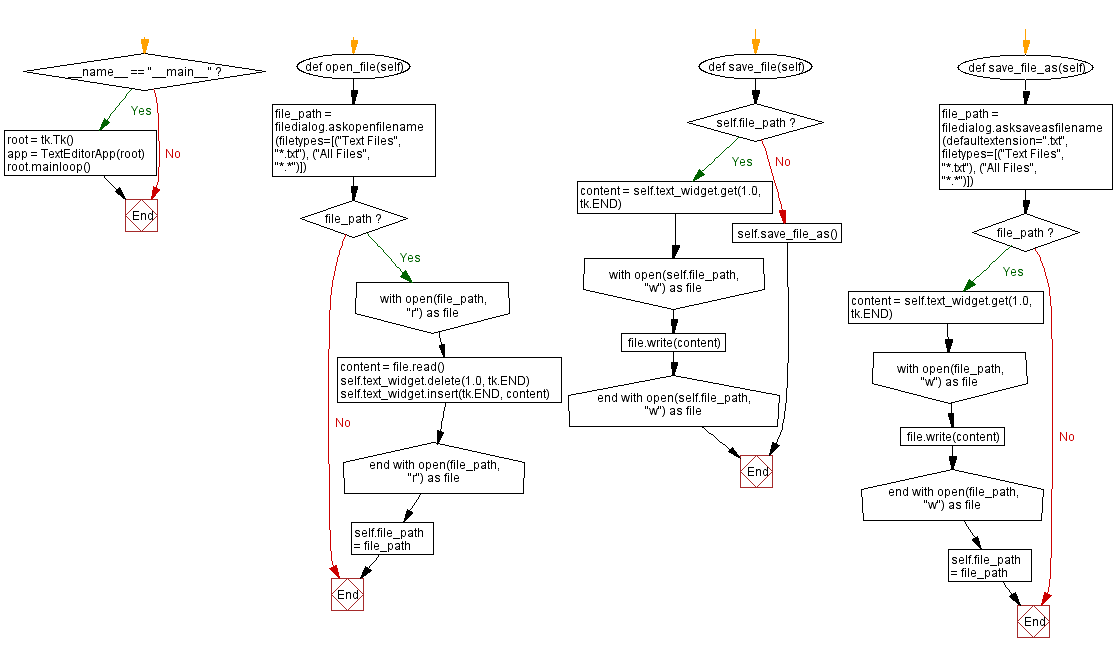
Python Code Editor:
Previous: Python Tkinter File Operations and Integration Home.
Next: Python Tkinter CSV viewer with tabular display.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics