Python HTTP Request with Network Exception Handling Example
Python urllib3 : Exercise-9 with Solution
Write a Python program that implements error handling for network-related exceptions that might occur during a request.
Sample Solution:
Python Code :
import urllib3
def make_http_request(url):
try:
# Create a PoolManager instance for managing HTTP connections
with urllib3.PoolManager() as http:
# Make an HTTP GET request
response = http.request('GET', url)
# Check if the request was successful (status code 200)
if response.status == 200:
print("Request Successful:")
print(response.data.decode('utf-8'))
else:
print(f"Error: Unable to fetch data. Status Code: {response.status}")
except urllib3.exceptions.RequestError as e:
print(f"Network Error: {e}")
except Exception as e:
print(f"Error: {e}")
if __name__ == "__main__":
# Define the URL for the HTTP request
target_url = 'https://www.example.com'
# Make the HTTP request with error handling
make_http_request(target_url)
Sample Output:
Request Successful: <!doctype html> <html> <head> <title>Example Domain</title> <meta charset="utf-8" /> <meta http-equiv="Content-type" content="text/html; charset=utf-8" /> <meta name="viewport" content="width=device-width, initial-scale=1" /> <style type="text/css"> body { background-color: #f0f0f2; margin: 0; padding: 0; font-family: -apple-system, system-ui, BlinkMacSystemFont, "Segoe UI", "Open Sans", "Helvetica Neue", Helvetica, Arial, sans-serif; } div { width: 600px; margin: 5em auto; padding: 2em; background-color: #fdfdff; border-radius: 0.5em; box-shadow: 2px 3px 7px 2px rgba(0,0,0,0.02); } a:link, a:visited { color: #38488f; text-decoration: none; } @media (max-width: 700px) { div { margin: 0 auto; width: auto; } } </style> </head> <body> <div> <h1>Example Domain</h1> <p>This domain is for use in illustrative examples in documents. You may use this domain in literature without prior coordination or asking for permission.</p> <p><a href="https://www.iana.org/domains/example">More information...</a></p> </div> </body> </html>
Note:
The warning we are seeing is an "InsecureRequestWarning" from the "urllib3" library, indicating that you are making an HTTPS request without SSL/TLS verification. This warning is raised because disabling SSL/TLS verification can expose your application to potential security risks, such as man-in-the-middle attacks.
Explanation:
Here's a brief explanation of the above Python urllib3 library code:
- Import Library:
- urllib3: Used for handling HTTP requests.
- Define Function:
- make_http_request(url): Makes an HTTP GET request to the specified URL with error handling for network-related exceptions.
- Make HTTP Request:
- Use urllib3.PoolManager() to manage HTTP connections.
- Inside the with block, make an HTTP GET request using http.request('GET', url).
- Check Response Status:
- Check if the response status is 200 (OK).
- If successful, print the response content.
- Error Handling:
- Use a try-except block to catch potential "urllib3" network-related exceptions (specifically, urllib3.exceptions.RequestError).
- If a network error occurs, print an appropriate error message.
- Additionally, catch the general "Exception" to handle unexpected errors and print an error message.
- Run the Function:
- Calls the make_http_request() function with the target URL to perform the HTTP request.
Flowchart:
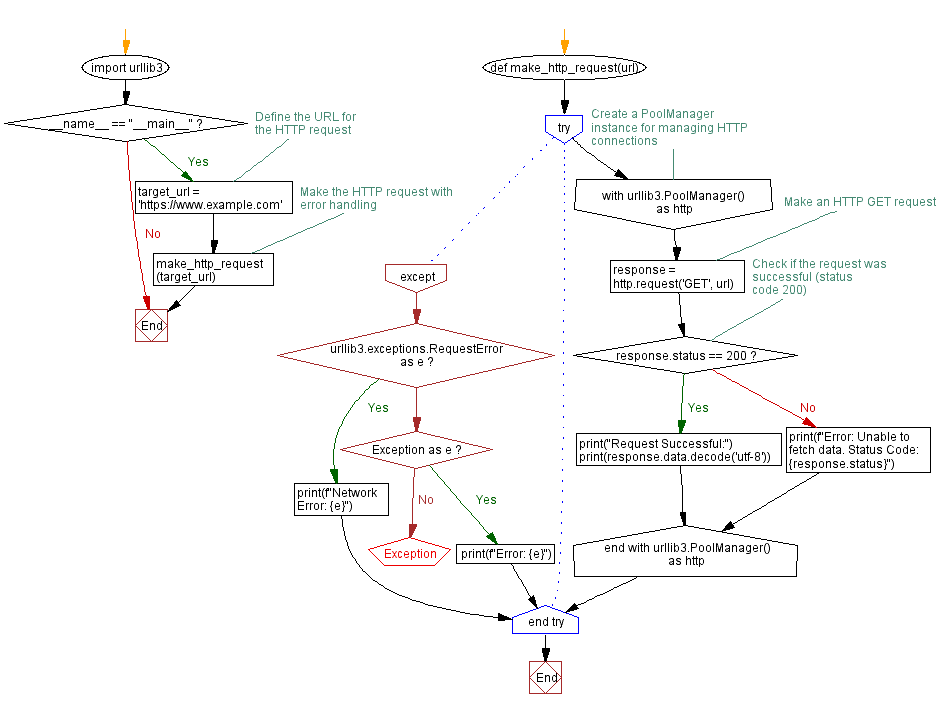
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Python HTTPS Requests with and without SSL/TLS verification example.
Next: Python Large File Download with Stream Parameter example.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics