Python HTTPS Requests with and without SSL/TLS verification example
Write a Python program that makes an HTTPS request with SSL/TLS verification enabled and then disables it to see the difference.
Sample Solution:
Python Code :
import urllib3
def make_https_request_with_verification():
# Define the HTTPS URL with SSL/TLS verification enabled
secure_url = 'https://www.example.com'
try:
# Create a PoolManager instance with SSL/TLS verification enabled
with urllib3.PoolManager() as http:
# Make an HTTPS GET request with SSL/TLS verification enabled
response = http.request('GET', secure_url)
# Check if the request was successful (status code 200)
if response.status == 200:
print("Secure HTTPS Request Successful:")
print(response.data.decode('utf-8'))
else:
print(f"Error: Unable to fetch data. Status Code: {response.status}")
except urllib3.exceptions.RequestError as e:
print(f"Error: {e}")
def make_https_request_without_verification():
# Define the HTTPS URL with SSL/TLS verification disabled
insecure_url = 'https://www.example.com'
try:
# Create a PoolManager instance with SSL/TLS verification disabled
with urllib3.PoolManager(cert_reqs='CERT_NONE') as http:
# Make an HTTPS GET request with SSL/TLS verification disabled
response = http.request('GET', insecure_url)
# Check if the request was successful (status code 200)
if response.status == 200:
print("Insecure HTTPS Request Successful:")
print(response.data.decode('utf-8'))
else:
print(f"Error: Unable to fetch data. Status Code: {response.status}")
except urllib3.exceptions.RequestError as e:
print(f"Error: {e}")
if __name__ == "__main__":
# Make an HTTPS request with SSL/TLS verification enabled
make_https_request_with_verification()
# Make an HTTPS request with SSL/TLS verification disabled
make_https_request_without_verification()
Sample Output:
Secure HTTPS Request Successful: <!doctype html> <html> <head> <title>Example Domain</title> <meta charset="utf-8" /> <meta http-equiv="Content-type" content="text/html; charset=utf-8" /> <meta name="viewport" content="width=device-width, initial-scale=1" /> <style type="text/css"> body { background-color: #f0f0f2; margin: 0; padding: 0; font-family: -apple-system, system-ui, BlinkMacSystemFont, "Segoe UI", "Open Sans", "Helvetica Neue", Helvetica, Arial, sans-serif; } div { width: 600px; margin: 5em auto; padding: 2em; background-color: #fdfdff; border-radius: 0.5em; box-shadow: 2px 3px 7px 2px rgba(0,0,0,0.02); } a:link, a:visited { color: #38488f; text-decoration: none; } @media (max-width: 700px) { div { margin: 0 auto; width: auto; } } </style> </head> <body> <div> <h1>Example Domain</h1> <p>This domain is for use in illustrative examples in documents. You may use this domain in literature without prior coordination or asking for permission.</p> <p><a href="https://www.iana.org/domains/example">More information...</a></p> </div> </body> </html> Insecure HTTPS Request Successful: <!doctype html> <html> <head> <title>Example Domain</title> <meta charset="utf-8" /> <meta http-equiv="Content-type" content="text/html; charset=utf-8" /> <meta name="viewport" content="width=device-width, initial-scale=1" /> <style type="text/css"> body { background-color: #f0f0f2; margin: 0; padding: 0; font-family: -apple-system, system-ui, BlinkMacSystemFont, "Segoe UI", "Open Sans", "Helvetica Neue", Helvetica, Arial, sans-serif; } div { width: 600px; margin: 5em auto; padding: 2em; background-color: #fdfdff; border-radius: 0.5em; box-shadow: 2px 3px 7px 2px rgba(0,0,0,0.02); } a:link, a:visited { color: #38488f; text-decoration: none; } @media (max-width: 700px) { div { margin: 0 auto; width: auto; } } </style> </head> <body> <div> <h1>Example Domain</h1> <p>This domain is for use in illustrative examples in documents. You may use this domain in literature without prior coordination or asking for permission.</p> <p><a href="https://www.iana.org/domains/example">More information...</a></p> </div> </body> </html> /usr/local/lib/python3.10/site-packages/urllib3/connectionpool.py:1056: InsecureRequestWarning: Unverified HTTPS request is being made to host 'www.example.com'. Adding certificate verification is strongly advised. See: https://urllib3.readthedocs.io/en/1.26.x/advanced-usage.html#ssl-warnings
Note:
The warning we are seeing is an "InsecureRequestWarning" from the "urllib3" library, indicating that you are making an HTTPS request without SSL/TLS verification. This warning is raised because disabling SSL/TLS verification can expose your application to potential security risks, such as man-in-the-middle attacks.
Explanation:
Here's a brief explanation of the above Python urllib3 library code:
- Import Library:
- urllib3: Used for handling HTTP requests.
- Define Functions:
- make_https_request_with_verification(): Makes an HTTPS request with SSL/TLS verification enabled.
- make_https_request_without_verification(): Makes an HTTPS request with SSL/TLS verification disabled.
- Make HTTPS Requests:
- Use urllib3.PoolManager() to manage HTTP connections.
- Make Secure HTTPS Request:
- In the make_https_request_with_verification() function:
- Creates a PoolManager instance with SSL/TLS verification enabled (cert_reqs set to the default value).
- Makes an HTTPS GET request to a secure URL with verification enabled.
- Checks the response status and prints the content if successful.
- Make Insecure HTTPS Request:
- In the make_https_request_without_verification() function:
- Creates a PoolManager instance with SSL/TLS verification disabled (cert_reqs set to 'CERT_NONE').
- Makes an HTTPS GET request to a secure URL with verification disabled.
- Checks the response status and prints the content if successful.
- Handle Exceptions:
- Uses a try-except block to catch potential "urllib3" request-related exceptions.
- Prints an error message if exceptions occur.
- Run the Functions:
- Calls both functions to make HTTPS requests with and without SSL/TLS verification.
Flowchart:
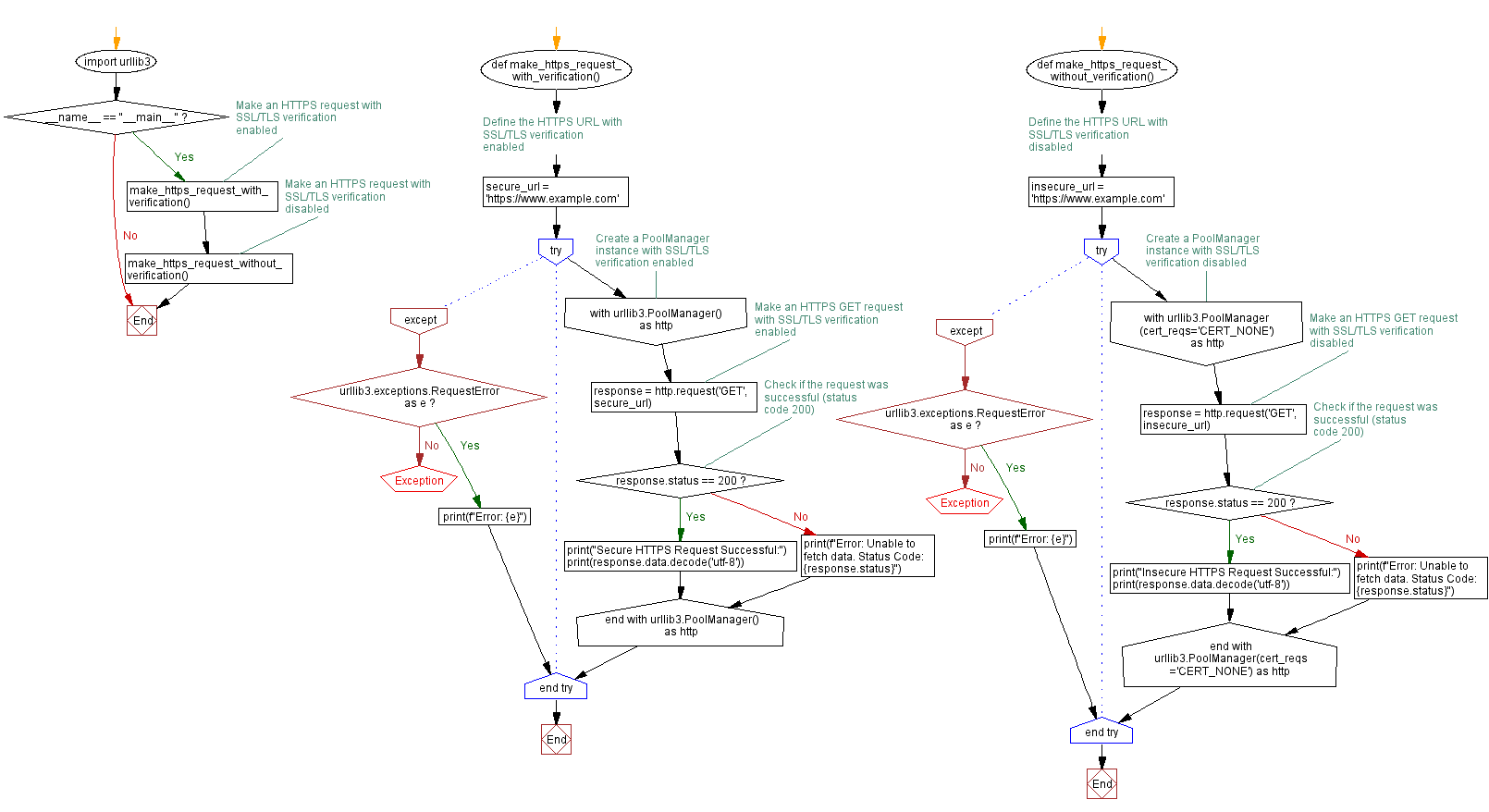
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Python Redirect Handling and final URL example.
Next: Write a Python program to create all possible strings by using 'a', 'e', 'i', 'o', 'u'. Use the characters exactly once.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.