C Exercises: Replace every element with the greatest element on its right side
C Array: Exercise-63 with Solution
Write a program in C to replace every element with the greatest element on its right side.
Expected Output :
The given array is : 7 5 8 9 6 8 5 7 4 6
After replace the modified array is: 9 9 9 8 8 7 7 6 6 0
The problem requires writing a C program to replace each element in an array with the greatest element among the elements to its right. The goal is to iterate through the array and for each element, find the maximum element to its right and replace the current element with this maximum. The last element of the array should be replaced with 0 as there are no elements to its right.
Visual Presentation:
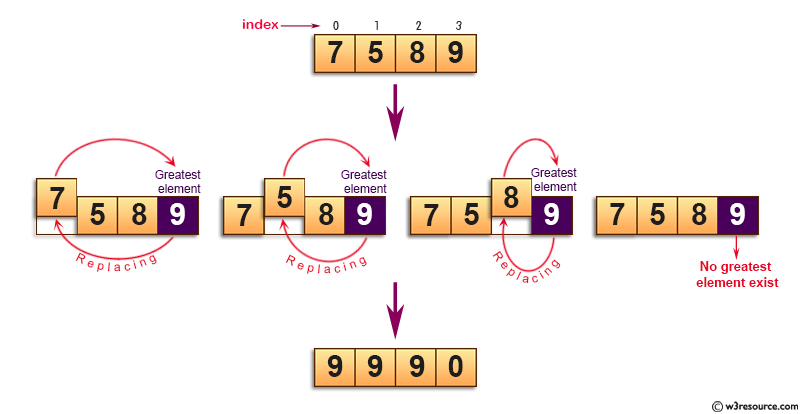
Sample Solution:
C Code:
#include <stdio.h>
// Function to print the elements of an array
void printArray(int a[], int n) {
for (int i = 0; i < n; i++)
printf("%d ", a[i]);
}
// Function to replace each element with the next greatest element
void replaceWithNextGreatest(int a[], int size) {
int maximum = a[size - 1]; // Initialize maximum as the last element
a[size - 1] = 0; // Update the last element to 0 as there is no greater element
// Iterate through the array from right to left
for (int i = size - 2; i >= 0; i--) {
int temp = a[i]; // Store the current element temporarily
a[i] = maximum; // Replace the current element with the maximum
if (maximum < temp)
maximum = temp; // Update maximum if the current element is greater
}
printf("After replacing, the modified array is: ");
printArray(a, size); // Print the modified array
}
int main() {
int i, arr1[] = {7, 5, 8, 9, 6, 8, 5, 7, 4, 6};
int n = sizeof(arr1) / sizeof(arr1[0]);
//------------- print original array ------------------
printf("The given array is : ");
for (i = 0; i < n; i++) {
printf("%d ", arr1[i]);
}
printf("\n");
//------------------------------------------------------
// Replace each element with the next greatest element
replaceWithNextGreatest(arr1, n);
return 0;
}
Sample Output:
The given array is : 7 5 8 9 6 8 5 7 4 6 After replace the modified array is: 9 9 9 8 8 7 7 6 6 0
Flowchart:
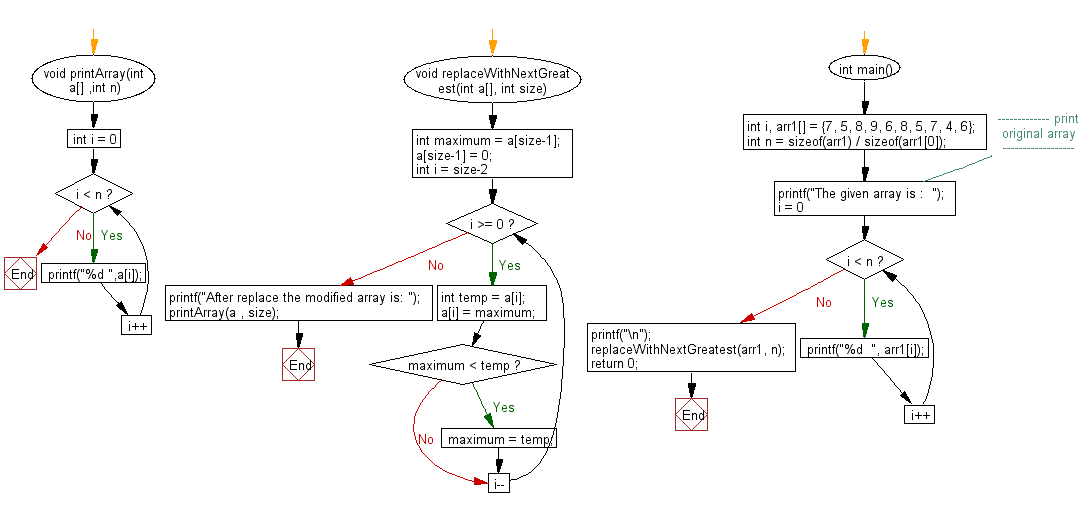
C Programming Code Editor:
Previous: Write a program in C to find the largest subarray with equal number of 0s and 1s.
Next: Write a program in C to find the median of two sorted arrays of same size.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics