C Exercises: Find largest number possible from the set of specified numbers
76. Largest Number from Given Set
Write a program in C to find the largest number possible from the set of given numbers.
Expected Output:
The given numbers are :
15 628 971 9 2143 12
The largest possible number by the given numbers are: 997162821431512
The problem involves writing a C program that arranges a set of given numbers to form the largest possible number. The program should take the individual numbers, determine the optimal order to concatenate them for the largest numerical value, and then display the resulting number. This requires comparing and sorting the numbers based on their potential contribution to the final combined value.
Visual Presentation:
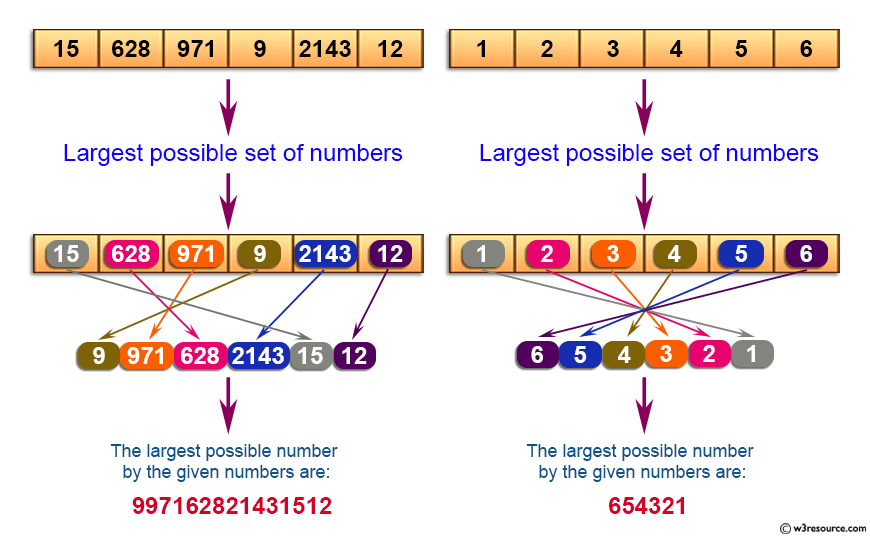
Sample Solution:
C Code:
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
// Comparison function for qsort to sort strings in a specific order to form the largest number
int compare(const void *a, const void *b) {
const char **X = (const char **)a;
const char **Y = (const char **)b;
// Determine the combined lengths of strings a and b, plus 1 for null terminator
int chr_len = strlen(*X) + strlen(*Y) + 1;
// Concatenate strings a and b in one order
char XY[chr_len];
strcpy(XY, *X);
strcat(XY, *Y);
// Concatenate strings b and a in another order
char YX[chr_len];
strcpy(YX, *Y);
strcat(YX, *X);
// Compare the concatenated strings to sort in descending order
return strcmp(YX, XY);
}
int main(void) {
// Array of strings
char *arr1[] = { "15", "628", "971", "9", "2143", "12" };
int n = sizeof(arr1) / sizeof(arr1[0]);
int i;
// Print original array
printf("The given numbers are : \n");
for (i = 0; i < n; i++) {
printf("%s ", arr1[i]);
}
printf("\n");
// Sort the array using qsort with custom comparison function
qsort(arr1, n, sizeof(arr1[0]), compare);
// Print the largest possible number formed by the given numbers
printf("The largest possible number by the given numbers are: ");
for (int i = 0; i < n; i++) {
printf("%s", arr1[i]);
}
return 0;
}
Output:
The given numbers are : 15 628 971 9 2143 12 The largest possible number by the given numbers are: 997162821431512
Flowchart:/p>
- Write a C program to form the largest possible number by concatenating an array of numbers using a custom comparator.
- Write a C program to arrange numbers to form the maximum possible numerical value without converting them to strings.
- Write a C program to generate all possible concatenations of numbers from an array and then choose the largest.
- Write a C program to rearrange numbers in an array using sorting by comparing string representations and then output the result.
C Programming Code Editor:
Previous: Write a program in C to find the sum of lower triangular elements of a matrix.
Next: Write a program in C to generate a random permutation of array elements.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.