C Exercises: Find the sum of lower triangular elements of a matrix
75. Sum of Lower Triangular Matrix Elements
Write a program in C to find the sum of the lower triangular elements of a matrix.
Expected Output:
The given array is :
1 2 3
4 5 6
7 8 9
The elements being summed of the lower triangular matrix are: 4 7 8
The Sum of the lower triangular Matrix Elements are: 19
The task is to write a C program that calculates the sum of the lower triangular elements of a given matrix. The lower triangular elements are those located on or below the main diagonal of the matrix. The program should traverse the matrix, identify these elements, compute their sum, and then display both the individual elements and their total sum.
Visual Presentation:
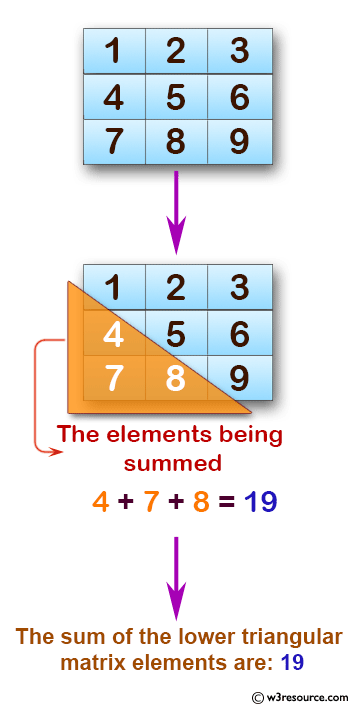
Sample Solution:
C Code:
#include <stdio.h>
int main() {
int R, C, n, r, c, sum = 0;
int arr1[3][3] = {{1, 2, 3},
{4, 5, 6},
{7, 8, 9}};
R = C = n = 3;
int i, j;
// Print original array
printf("The given array is : \n");
for (i = 0; i < R; i++) {
for (j = 0; j < C; j++) {
printf("%d ", arr1[i][j]);
}
printf("\n");
}
printf("The elements being summed of the lower triangular matrix are: ");
for (r = 0; r < R; r++) {
for (c = 0; c < C; c++) {
if (r > c) {
printf("%d ", arr1[r][c]); // Print the elements of the lower triangular matrix
sum += arr1[r][c]; // Add the elements of the lower triangular matrix
}
}
}
printf("\nThe Sum of the lower triangular Matrix Elements are: %d", sum);
return 0;
}
Output:
The given array is : 1 2 3 4 5 6 7 8 9 The elements being summed of the lower triangular matrix are: 4 7 8 The Sum of the lower triangular Matrix Elements are: 19
Flowchart:/p>
For more Practice: Solve these Related Problems:
- Write a C program to compute the sum of the lower triangular portion of a matrix using nested loops.
- Write a C program to extract lower triangular elements into a separate array and compute their sum.
- Write a C program to calculate the lower triangular sum using pointer arithmetic and recursion.
- Write a C program to compute and print the lower triangular matrix elements and then their cumulative sum.
C Programming Code Editor:
Previous: Write a program in C to find the sum of upper triangular elements of a matrix.
Next: Write a program in C to find largest number possible from the set of given numbers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.