C Exercises: Find the sum of upper triangular elements of a matrix
74. Sum of Upper Triangular Matrix Elements
Write a program in C to find the sum of the upper triangular elements of a matrix.
Expected Output:
The given array is :
1 2 3
4 5 6
7 8 9
The elements being summed of the upper triangular matrix are: 2 3 6
The Sum of the upper triangular Matrix Elements are: 11
The task is to write a C program that computes the sum of the upper triangular elements of a given matrix. The upper triangular elements are those located on or above the main diagonal of the matrix. The program should iterate through the matrix, identify these elements, calculate their sum, and then display both the elements and their total sum.
Visual Presentation:
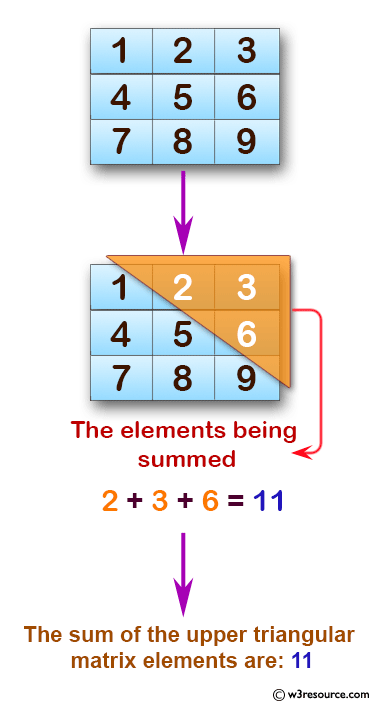
Sample Solution:
C Code:
#include <stdio.h>
int main() {
int R, C, n, r, c, sum = 0;
int arr1[3][3] = {{1, 2, 3},
{4, 5, 6},
{7, 8, 9}};
R = C = n = 3;
int i, j;
// Print original array
printf("The given array is : \n");
for (i = 0; i < R; i++) {
for (j = 0; j < C; j++) {
printf("%d ", arr1[i][j]);
}
printf("\n");
}
printf("The elements being summed of the upper triangular matrix are: ");
for (r = 0; r < R; r++) {
for (c = 0; c < C; c++) {
if (r < c) {
printf("%d ", arr1[r][c]);
sum += arr1[r][c]; // Add the elements of the upper triangular matrix
}
}
}
printf("\nThe Sum of the upper triangular Matrix Elements are: %d", sum);
return 0;
}
Output:
The given array is : 1 2 3 4 5 6 7 8 9 The elements being summed of the upper triangular matrix are: 2 3 6 The Sum of the upper triangular Matrix Elements are: 11
For more Practice: Solve these Related Problems:
- Write a C program to compute the sum of the upper triangular part of a matrix using nested loops.
- Write a C program to extract the upper triangular elements into a new array and then sum them.
- Write a C program to calculate the sum of upper triangular elements using pointer arithmetic on a 2D array.
- Write a C program to compute the upper triangular sum and then display the average of these elements.
C Programming Code Editor:
Previous: Write a program in C to print all unique elements of an unsorted array.
Next: Write a program in C to find the sum of lower triangular elements of a matrix.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.