C Exercises: Print all unique elements of an unsorted array
C Array: Exercise-73 with Solution
Write a program in C to print all unique elements of an unsorted array.
Expected Output:
The given array is : 1 5 8 5 7 3 2 4 1 6 2
Unique Elements in the given array are:
1 5 8 7 3 2 4 6
The problem requires writing a C program to identify and print all unique elements from an unsorted array. The program should efficiently determine which elements appear only once in the array and then display these unique elements in the order of their first occurrence.
Sample Solution:
C Code:
#include <stdio.h>
int main() {
int arr1[] = {1, 5, 8, 5, 7, 3, 2, 4, 1, 6, 2};
int n = sizeof(arr1) / sizeof(int);
int i, j;
// Print the original array
printf("The given array is : ");
for (i = 0; i < n; i++) {
printf("%d ", arr1[i]);
}
printf("\n");
printf("Unique Elements in the given array are: \n");
for (i = 0; i < n; i++) {
// Iterate through the array to find unique elements
for (j = 0; j < i; j++) {
// If a duplicate is found, break the loop
if (arr1[i] == arr1[j])
break;
}
// If 'i' reaches 'j', the current element is unique, hence print it
if (i == j) {
printf("%d ", arr1[i]);
}
}
return 0;
}
Output:
The given array is : 1 5 8 5 7 3 2 4 1 6 2 Unique Elements in the given array are: 1 5 8 7 3 2 4 6
Flowchart:
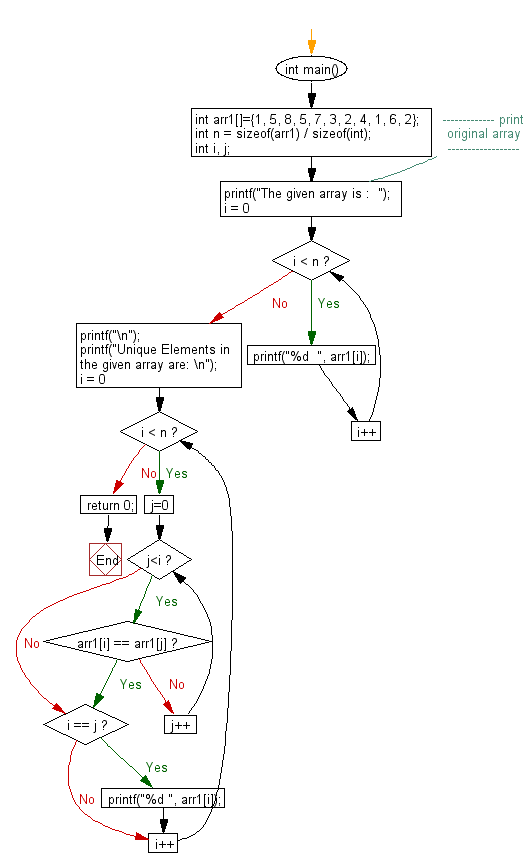
C Programming Code Editor:
Previous: Write a program in C to return only the unique rows from a given binary matrix.
Next: Write a program in C to find the sum of upper triangular elements of a matrix.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics