C Exercises: Find the maximum repeating number in a given array
C Array: Exercise-81 with Solution
Write a program in C to find the maximum repeating number in a given array.
The array range is [0..n-1] and the elements are in the range [0..k-1] and k<=n.
Expected Output:
The given array is:
2 3 3 5 3 4 1 7 7 7 7
The maximum repeating number is: 7
The task is to write a C program to find the maximum repeating number in a given array where the array length is n and the elements are within the range [0, k-1], with k<= n. The program should iterate through the array to count the occurrences of each number and identify the number that appears the most frequently. The output should display this maximum repeating number.
Sample Solution:
C Code:
#include <stdio.h>
// Function to find the number with maximum repetition
int numToRepeatMax(int* arr1 , int n, int k) {
int mx = arr1[0], result = 0;
// Adjusting the elements in the array to identify the maximum repeating element
for (int i = 0; i < n; i++)
arr1[arr1[i] % k] += k;
// Finding the number with the maximum repetition
for (int i = 1; i < n; i++) {
if (arr1[i] > mx) {
mx = arr1[i];
result = i;
}
}
return result;
}
int main() {
int arr1[] = {2, 3, 3, 5, 3, 4, 1, 7, 7, 7, 7};
int n = sizeof(arr1) / sizeof(arr1[0]);
int i;
// Printing the original array
printf("The given array is: \n");
for (i = 0; i < n; i++) {
printf("%d ", arr1[i]);
}
printf("\n");
int k = 8; // Given value 'k'
printf("The maximum repeating number is: %d", numToRepeatMax(arr1, n, k)); // Finding and printing the number with maximum repetition
return 0;
}
Output:
The given array is: 2 3 3 5 3 4 1 7 7 7 7 The maximum repeating number is: 7
Flowchart:
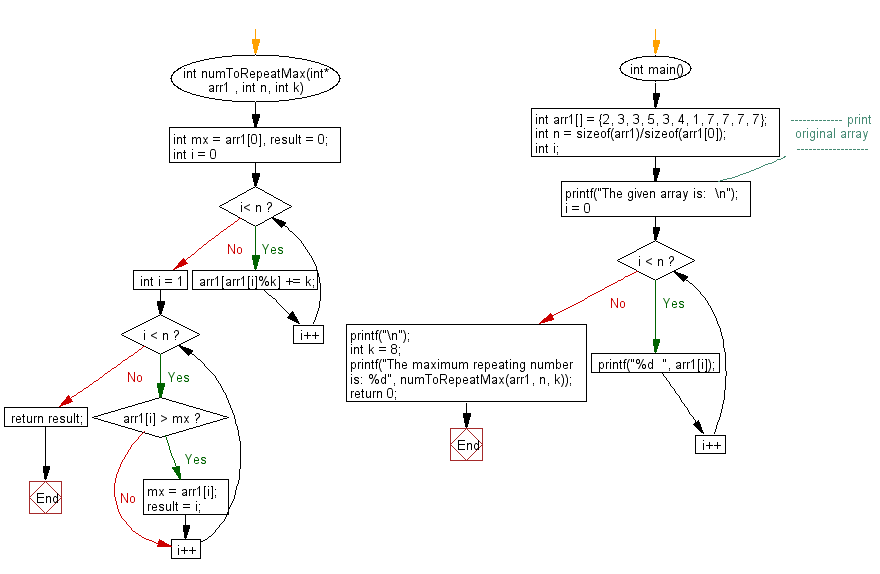
C Programming Code Editor:
Previous: Write a program in C to count all distinct pairs for a specific difference.
Next: Write a program in C to print all possible combinations of r elements in a given array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics