C Exercises: Print all possible combinations of r elements in a given array
82. Combinations of r Elements from Array
Write a program in C to print all possible combinations of r elements in a given array.
Expected Output:
The given array is:
1 5 4 6 8 The combination from by the number of elements are: 3
The combinations are:
1 5 4 6
1 5 4 8
1 5 6 8
1 4 6 8
5 4 6 8
The task is to write a C program that prints all possible combinations of r elements from a given array. The program should generate and display each unique combination of the specified number of elements. The output should list all the possible combinations formed from the array elements for the given value of r.
Sample Solution:
C Code:
#include <stdio.h>
// Function to generate combinations
void makeCombination(int arr1[], int data[], int st, int end, int index, int r);
// Function to display combinations
void CombinationDisplay(int arr1[], int n, int r) {
int data[r]; // Temporary array to store the combination
makeCombination(arr1, data, 0, n - 1, 0, r); // Generate combinations
}
// Recursive function to generate combinations
void makeCombination(int arr1[], int data[], int st, int end, int index, int r) {
// Base case: if index equals r, print the combination
if (index == r) {
for (int j = 0; j < r; j++)
printf("%d ", data[j]); // Print the combination elements
printf("\n"); // Move to the next line for the next combination
return;
}
// Generate combinations recursively
for (int i = st; i <= end && end - i + 1 >= r - index; i++) {
data[index] = arr1[i]; // Fill data[] with elements of arr1[] for the current combination
makeCombination(arr1, data, i + 1, end, index + 1, r); // Recursively generate combinations
}
}
int main() {
int arr1[] = {1, 5, 4, 6, 8};
int r = 4; // Number of elements in each combination
int n = sizeof(arr1) / sizeof(arr1[0]); // Calculate array size
int i;
// Printing the original array
printf("The given array is: \n");
for (i = 0; i < n; i++) {
printf("%d ", arr1[i]);
}
printf("\n");
printf("The combination from by the number of elements are: %d\n", r);
printf("The combinations are: \n");
CombinationDisplay(arr1, n, r); // Display combinations
return 0;
}
Output:
The given array is: 1 5 4 6 8 The combination from by the number of elements are: 4 The combinations are: 1 5 4 6 1 5 4 8 1 5 6 8 1 4 6 8 5 4 6 8
Visual Presentation:
Flowchart 1:
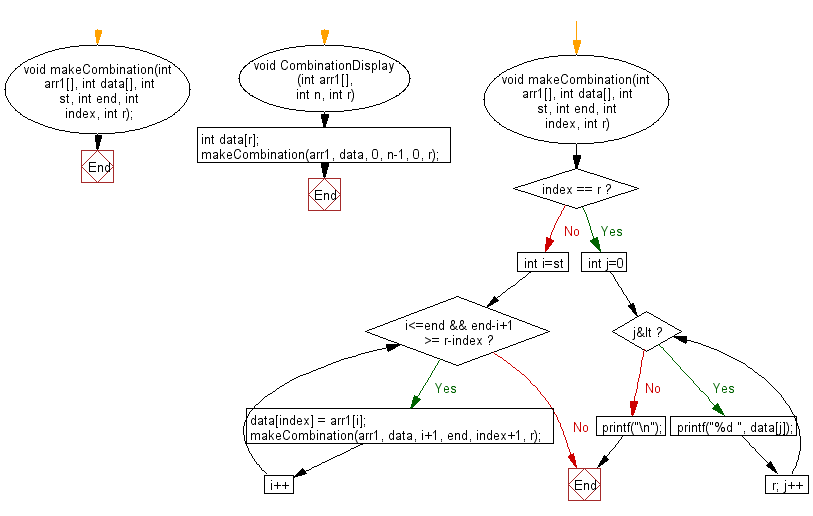
Flowchart 2:
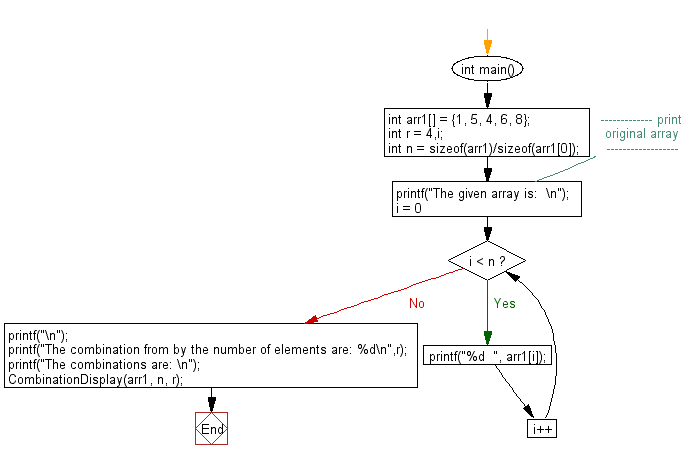
For more Practice: Solve these Related Problems:
- Write a C program to generate all possible combinations of r elements from a given array using recursion.
- Write a C program to print all combinations of r elements in lexicographical order.
- Write a C program to generate combinations and then count the total number of combinations.
- Write a C program to generate and store all r-element combinations in a dynamically allocated 2D array.
C Programming Code Editor:
Previous: Write a program in C to find the maximum repeating number in a given array.The array range is [0..n-1] and the elements are in the range [0..k-1] and k<=n.
Next: Write a program in C to find a pair with the given difference.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.