C Exercises: Find a pair with the given difference
83. Find a Pair with Given Difference
Write a program in C to find a pair with the given difference.
Expected Output:
The given array is:
1 15 39 75 92
The given difference is: 53
The pair are: (39, 92)
The task is to write a C program to find a pair of elements in a given array that have a specific difference. The program should iterate through the array to identify and display the pair of numbers whose absolute difference matches the given value. The output should display the pair that meets this condition.
Sample Solution:
C Code:
#include <stdio.h>
#include <stdbool.h>
// Function to find the pair with the given difference
bool pairFinding(int arr1[], int size, int d) {
int i = 0;
int j = 1;
while (i < size && j < size) {
if (i != j && arr1[j] - arr1[i] == d) {
printf("The pair are: (%d, %d)", arr1[i], arr1[j]); // Print the pair
return true;
} else if (arr1[j] - arr1[i] < d) {
j++; // Increment j to check the next element
} else {
i++; // Increment i to check the next element
}
}
printf("No such pair found in the given array."); // Print if no pair found
return false;
}
int main() {
int arr1[] = {1, 15, 39, 75, 92};
int size = sizeof(arr1) / sizeof(arr1[0]); // Calculate array size
int d = 53;
int i;
// Printing the original array
printf("The given array is: \n");
for(i = 0; i < size; i++) {
printf("%d ", arr1[i]);
}
printf("\n");
printf("The given difference is: %d\n", d);
pairFinding(arr1, size, d); // Function call to find the pair with the given difference
return 0;
}
Output:
The given array is: 1 15 39 75 92 The given difference is: 53 The pair are: (39, 92)
Visual Presentation:
Flowchart 1:
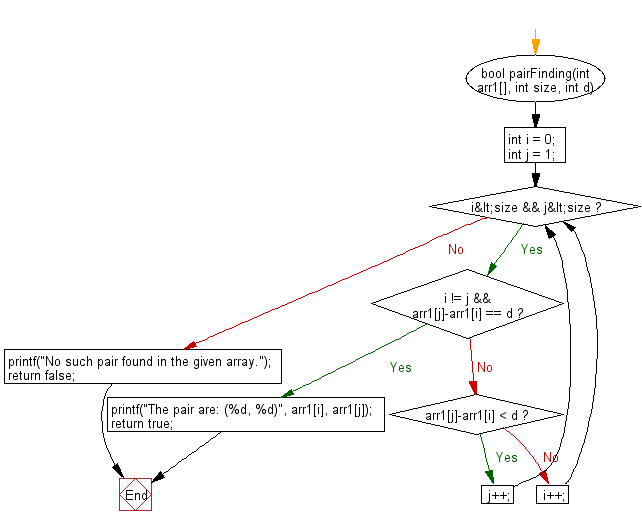
Flowchart 2:
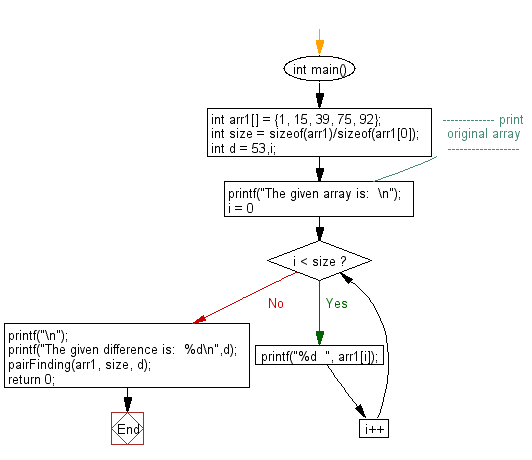
For more Practice: Solve these Related Problems:
- Write a C program to find a pair of numbers with a given difference using a hash table for O(n) complexity.
- Write a C program to search for a pair with the given difference in a sorted array using two-pointer technique.
- Write a C program to find all pairs with a given difference using nested loops and then eliminate duplicate pairs.
- Write a C program to find a pair with a given difference recursively after sorting the array.
C Programming Code Editor:
Previous: Write a program in C to print all possible combinations of r elements in a given array.
Next: Write a program in C to find the minimum distance between two numbers in a given array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.