C Exercises: Find the index of first peak element in a given array
C Array: Exercise-97 with Solution
Write a program in C to find the index of the first peak element in a given array.
Expected Output:
The given array is:
5 12 13 20 16 19 11 7 25
The index of first peak element in the array is: 3
The task is to write a C program that finds the index of the first peak element in a given array. A peak element is defined as an element that is greater than its neighbors. The program should traverse the array, identify the first occurrence of such an element, and display its index.
Sample Solution:
C Code:
#include<stdio.h>
// Function to search for a peak element recursively
int peakElementSearch(int arr1[], int ele_low, int ele_high, int n)
{
int ele_mid = ele_low + (ele_high - ele_low) / 2;
// Check if the element at mid is a peak or not
if ((ele_mid == 0 || arr1[ele_mid - 1] <= arr1[ele_mid]) &&
(ele_mid == n - 1 || arr1[ele_mid + 1] <= arr1[ele_mid]))
return ele_mid;
else if (ele_mid > 0 && arr1[ele_mid - 1] > arr1[ele_mid])
return peakElementSearch(arr1, ele_low, (ele_mid - 1), n);
else
return peakElementSearch(arr1, (ele_mid + 1), ele_high, n);
}
// Function to find a peak element in an array
int PeakFinding(int arr1[], int n)
{
return peakElementSearch(arr1, 0, n - 1, n);
}
int main()
{
int arr1[] = {5, 12, 13, 20, 16, 19, 11, 7, 25};
int n = sizeof(arr1) / sizeof(arr1[0]);
int i = 0;
// Print the original array
printf("The given array is: \n");
for (i = 0; i < n; i++)
{
printf("%d ", arr1[i]);
}
printf("\n");
// Find the index of the first peak element in the array
printf("The index of the first peak element in the array is: %d", PeakFinding(arr1, n));
return 0;
}
Output:
The given array is: 5 12 13 20 16 19 11 7 25 The index of first peak element in the array is: 3
Visual Presentation:
Flowchart:
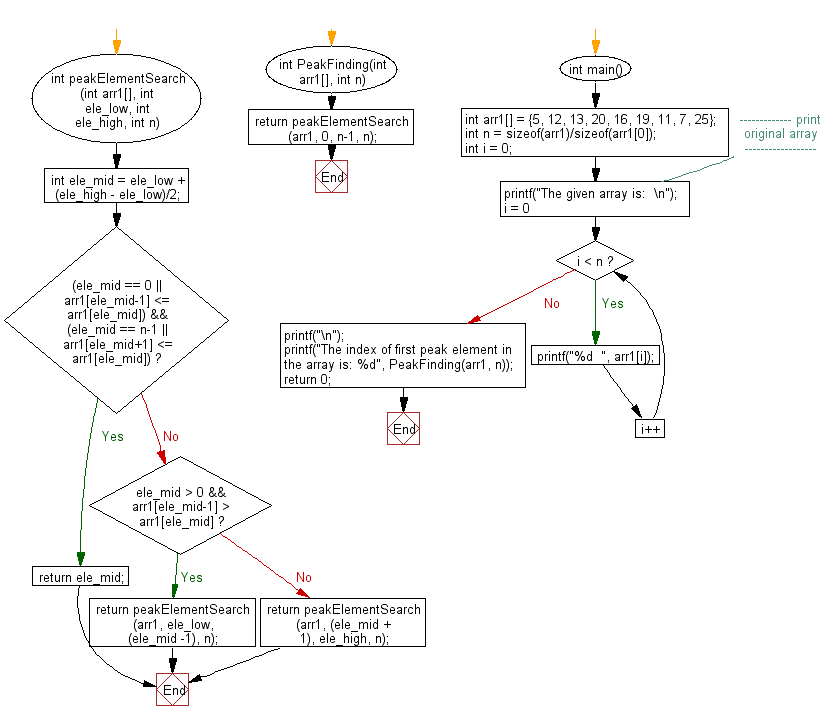
C Programming Code Editor:
Previous: Write a program in C to segregate even and odd elements on an array.
Next: Write a program in C to return the largest span found in the leftmost and rightmost appearances of same value(values are inclusive) in a given array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics