C Exercises: Segregate even and odd elements on an array
C Array: Exercise-96 with Solution
Write a program in C to segregate even and odd elements in an array.
Expected Output:
The given array is:
17 42 19 7 27 24 30 54 73
The array after segregation is: 54 42 30 24 27 7 19 17 73
The task is to write a C program that segregates even and odd elements in a given array, such that all even elements are moved to the beginning and all odd elements to the end of the array. The program should iterate through the array, rearrange the elements accordingly, and then display the modified array with all even numbers followed by all odd numbers.
Sample Solution:
C Code:
#include<stdio.h>
// Function to swap two elements
void changePlace(int *ar, int *br)
{
int temp = *ar;
*ar = *br;
*br = temp;
}
// Function to segregate even and odd numbers in an array
void EvenOddSegre(int arr1[], int size)
{
int l_index = 0, r_index = size - 1;
// Segregating even and odd numbers
while (l_index < r_index)
{
// Move left_index until an odd number is found
while (arr1[l_index] % 2 == 0 && l_index < r_index)
l_index++;
// Move right_index until an even number is found
while (arr1[r_index] % 2 == 1 && l_index < r_index)
r_index--;
// Swap the found odd and even elements
if (l_index < r_index)
{
changePlace(&arr1[l_index], &arr1[r_index]);
l_index++;
r_index--;
}
}
}
int main()
{
int arr1[] = {17, 42, 19, 7, 27, 24, 30, 54, 73};
int arr_size = sizeof(arr1) / sizeof(arr1[0]);
int i = 0;
// Print the original array
printf("The given array is: \n");
for(i = 0; i < arr_size; i++)
{
printf("%d ", arr1[i]);
}
printf("\n");
// Segregate even and odd numbers
EvenOddSegre(arr1, arr_size);
// Print the segregated array
printf("The array after segregation is: ");
for (i = 0; i < arr_size; i++)
printf("%d ", arr1[i]);
return 0;
}
Output:
The given array is: 17 42 19 7 27 24 30 54 73 The array after segregation is: 54 42 30 24 27 7 19 17 73
Visual Presentation:
Flowchart:
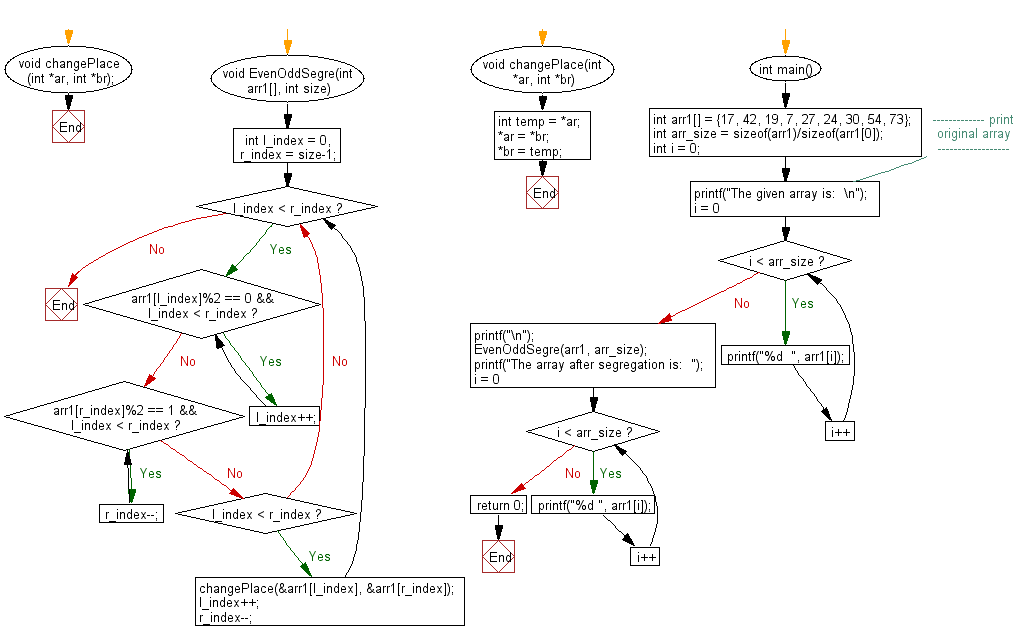
C Programming Code Editor:
Previous: Write a program in C to segregate 0s and 1s in an array.
Next: Write a program in C to find the index of first peak element in a given array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/c-programming-exercises/array/c-array-exercise-96.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics