C Exercises: Segregate 0s and 1s in an array
C Array: Exercise-95 with Solution
Write a program in C to segregate 0s and 1s in an array.
Expected Output:
The given array is:
1 0 1 0 0 1 0 1 1<>br>
The array after segregation is: 0 0 0 0 1 1 1 1 1
The task is to write a C program that segregates 0s and 1s in a given array such that all 0s are moved to the beginning and all 1s to the end of the array. The program should traverse the array, rearrange the elements accordingly, and then display the modified array with all 0s followed by all 1s.
Sample Solution:
C Code:
#include <stdio.h>
// Function to segregate 0s and 1s in an array
void segZeroAndOne(int arr1[], int n)
{
int ctr = 0; // Counter to keep track of 0s
// Count the number of 0s in the array
for (int i = 0; i < n; i++) {
if (arr1[i] == 0)
ctr++;
}
// Assign 0s to the first 'ctr' elements of the array
for (int i = 0; i < ctr; i++)
arr1[i] = 0;
// Assign 1s to the remaining elements of the array
for (int i = ctr; i < n; i++)
arr1[i] = 1;
}
// Function to print the segregated array
void printSegre(int arr1[], int n)
{
printf("The array after segregation is: ");
for (int i = 0; i < n; i++)
printf("%d ", arr1[i]);
}
int main()
{
// Given input array
int arr1[] = { 1, 0, 1, 0, 0, 1, 0, 1, 1 };
int n = sizeof(arr1) / sizeof(arr1[0]);
int i;
//------------- print original array ------------------
printf("The given array is: \n");
for(i = 0; i < n; i++)
{
printf("%d ", arr1[i]); // Print the original array
}
printf("\n");
//-----------------------------------------------------------
// Segregate 0s and 1s in the array
segZeroAndOne(arr1, n);
// Print the segregated array
printSegre(arr1, n);
return 0;
}
Output:
The given array is: 1 0 1 0 0 1 0 1 1 The array after segregation is: 0 0 0 0 1 1 1 1 1
Visual Presentation:
Flowchart:
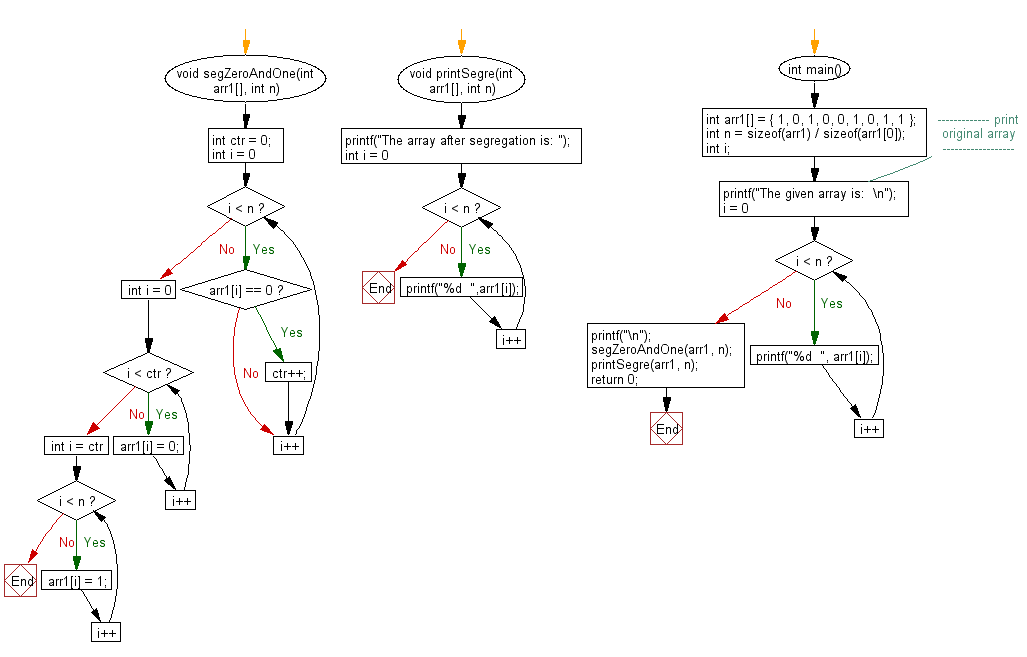
C Programming Code Editor:
Previous: Write a program in C to find the maximum for each and every contigious subarray of size k from a given array.
Next: Write a program in C to segregate even and odd elements on an array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/c-programming-exercises/array/c-array-exercise-95.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics