C Exercises: Check two given integers, and return true if one of them is 30 or if their sum is 30
3. Check if One or Sum is 30
Write a C program that checks two given integers and returns true if at least one of them is 30 or if their sum is 30. In other words, if either of the two integers is 30 or if their sum equals 30, the program will return true.
C Code:
#include <stdio.h> // Include standard input/output library
int test(int x, int y); // Declare the function 'test' with two integer parameters
int main(void)
{
// Call the function 'test' with arguments 25 and 5, and print the result
printf("%d", test(25, 5));
// Print a newline for formatting
printf("\n");
// Call the function 'test' with arguments 20 and 30, and print the result
printf("%d", test(20, 30));
// Print a newline for formatting
printf("\n");
// Call the function 'test' with arguments 20 and 25, and print the result
printf("%d", test(20, 25));
}
// Function definition for 'test'
int test(int x, int y)
{
// Return 1 (true) if any of the following conditions are true:
// 1. x is equal to 30
// 2. y is equal to 30
// 3. the sum of x and y is equal to 30
return x == 30 || y == 30 || (x + y == 30);
}
Sample Output:
1 1 0
Explanation:
int test(int x, int y) { return x == 30 || y == 30 || (x + y == 30); }
The function test takes two integer arguments x and y. It checks if either of the arguments is equal to 30 or if their sum is equal to 30. If any of these conditions are true, it returns 1, otherwise it returns 0.
Time complexity and space complexity:
Time complexity: The function only performs a few arithmetic and comparison operations, so the time complexity is constant O(1).
Space complexity: The function only uses a few variables to store the arguments and the result, so the space complexity is also constant O(1).
Pictorial Presentation:
Flowchart:
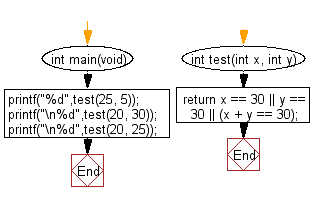
For more Practice: Solve these Related Problems:
- Write a C program that checks if one of two given numbers is 50 or if their sum is 50.
- Write a C program that checks if one of three given numbers is 40 or if their product is 40.
- Write a C program that checks if one of two given numbers is a multiple of 7 or if their sum is a multiple of 7.
- Write a C program that checks if the sum of two numbers is a perfect square.
C Programming Code Editor:
Previous: Write a C program to get the absolute difference between n and 51. If n is greater than 51 return triple the absolute difference.
Next: Write a C program to check a given integer and return true if it is within 10 of 100 or 200.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.