C Exercises: Check a given integer and return true if it is within 10 of 100 or 200
C-programming basic algorithm: Exercise-4 with Solution
Write a C program to check a given integer and return true if it is within 10 of 100 or 200.
C Code:
#include <stdio.h> // Include standard input/output library
#include <stdlib.h> // Include standard library for absolute value function
int test(int x); // Declare the function 'test' with an integer parameter
int main(void)
{
// Call the function 'test' with argument 103 and print the result
printf("%d", test(103));
// Print a newline for formatting
printf("\n");
// Call the function 'test' with argument 90 and print the result
printf("%d", test(90));
// Print a newline for formatting
printf("\n");
// Call the function 'test' with argument 89 and print the result
printf("%d", test(89));
}
// Function definition for 'test'
int test(int x)
{
// Check if the absolute difference between 'x' and 100 is less than or equal to 10,
// or if the absolute difference between 'x' and 200 is less than or equal to 10.
// Return 1 (true) if either condition is true, otherwise return 0 (false).
if (abs(x - 100) <= 10 || abs(x - 200) <= 10)
return 1;
return 0;
}
Sample Output:
1 1 0
Explanation:
int test(int x) { if (abs(x - 100) <= 10 || abs(x - 200) <= 10) return 1; return 0; }
The above function ‘test’ takes an integer ‘x’ as input and checks whether the absolute difference between ‘x’ and 100 or ‘x’ and 200 is less than or equal to 10. If either of these conditions is true, the function returns 1, otherwise it returns 0.
Time complexity and space complexity:
The time complexity of this function is O(1) because the code executes a fixed number of operations, regardless of the size of the input.
The space complexity of this function is O(1) because it only uses a fixed amount of memory, regardless of the size of the input.
Flowchart:
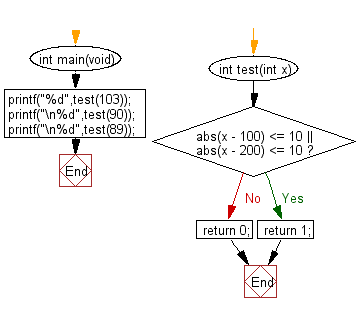
C Programming Code Editor:
Previous: Write a C program to check two given integers, and return true if one of them is 30 or if their sum is 30.
Next: Write a C program to check if a given positive number is a multiple of 3 or a multiple of 7.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/c-programming-exercises/basic-algo/c-programming-basic-algorithm-exercises-4.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics