C Exercises: Check whether a given positive number is a multiple of 3 or a multiple of 7
5. Divisibility by 3 or 7
Write a C program that checks if a positive integer is divisible by either 3 or 7, or both. If the integer is a multiple of 3, then the program will return true. Similarly, if the integer is a multiple of 7, then also the program will return true. If the integer is not a multiple of 3 or 7, then the program will return false.
C Code:
#include <stdio.h> // Include standard input/output library
#include <stdlib.h> // Include standard library for modulo operation
int test(int n); // Declare the function 'test' with an integer parameter
int main(void)
{
// Call the function 'test' with argument 3 and print the result
printf("%d", test(3));
// Print a newline for formatting
printf("\n");
// Call the function 'test' with argument 14 and print the result
printf("%d", test(14));
// Print a newline for formatting
printf("\n");
// Call the function 'test' with argument 12 and print the result
printf("%d", test(12));
// Print a newline for formatting
printf("\n");
// Call the function 'test' with argument 37 and print the result
printf("%d", test(37));
}
// Function definition for 'test'
int test(int n)
{
// Check if 'n' is divisible by 3 or if 'n' is divisible by 7.
// Return 1 (true) if either condition is true, otherwise return 0 (false).
return n % 3 == 0 || n % 7 == 0;
}
Sample Output:
1 1 1 0
Explanation:
int test(int n) { return n % 3 == 0 || n % 7 == 0; }
This function ‘test’ takes an integer (n) as input and checks if it is divisible by 3 or 7 or not. If it is divisible by either 3 or 7, then it returns 1, otherwise, it returns 0.
Time complexity and space complexity:
Time complexity: O(1) - the function has a constant time complexity, as it performs a single arithmetic operation and a few conditional checks, which take a constant amount of time.
Space complexity: O(1) - the function uses a fixed amount of memory to store the input integer and the boolean value it returns, which does not depend on the size of the input.
Pictorial Presentation:
Flowchart:
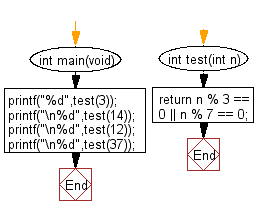
For more Practice: Solve these Related Problems:
- Write a C program to check if a positive integer is divisible by 5 or 11, but not both.
- Write a C program that returns true if a given integer is divisible by 4 or 6, and false otherwise.
- Write a C program to check if a given number is divisible by either 2, 3, or 5, but only one of them.
- Write a C program to determine if a number is a multiple of 3, and if so, check if it is also a multiple of 9.
C Programming Code Editor:
Previous: Write a C program to check a given integer and return true if it is within 10 of 100 or 200.
Next: Write a C program to check whether a given temperatures is less than 0 and the other is greater than 100.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.