C Exercises: Check whether a given temperatures is less than 0 and the other is greater than 100
6. Temperature Range Check
Write a C program that checks two given temperatures and returns true if one temperature is less than 0 and the other is greater than 100, otherwise it returns false.
C Code:
#include <stdio.h> // Include standard input/output library
#include <stdlib.h> // Include standard library for absolute value function
int test(int temp1, int temp2); // Declare the function 'test' with two integer parameters
int main(void)
{
// Call the function 'test' with arguments 120 and -1, and print the result
printf("%d", test(120, -1));
// Print a newline for formatting
printf("\n");
// Call the function 'test' with arguments -1 and 120, and print the result
printf("%d", test(-1, 120));
// Print a newline for formatting
printf("\n");
// Call the function 'test' with arguments 2 and 120, and print the result
printf("%d", test(2, 120));
}
// Function definition for 'test'
int test(int temp1, int temp2)
{
// Check if either of the following conditions are true:
// 1. temp1 is less than 0 and temp2 is greater than 100
// 2. temp2 is less than 0 and temp1 is greater than 100
// Return 1 (true) if either condition is true, otherwise return 0 (false).
return temp1 < 0 && temp2 > 100 || temp2 < 0 && temp1 > 100;
}
Sample Output:
1 1 0
Explanation:
int test(int temp1, int temp2) { return temp1 < 0 && temp2 > 100 || temp2 < 0 && temp1 > 100; }
The above function ‘test’ takes in two integer arguments ‘temp1’ and ‘temp2’. It returns 1 (true) if ‘temp1’ is negative and ‘temp2’ is greater than 100, or if ‘temp2’ is negative and ‘temp1’ is greater than 100, else it returns 0 (false).
Time complexity and space complexity:
The time complexity of the function is O(1), which means it takes a constant amount of time to execute, regardless of the input size.
The space complexity of the function is O(1), as it only uses a constant amount of memory, regardless of the input size.
Pictorial Presentation:
Flowchart:
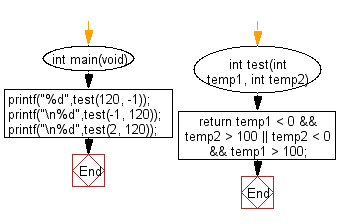
For more Practice: Solve these Related Problems:
- Write a C program that checks two given temperatures and returns true if one is below -20 and the other above 80.
- Write a C program to check if one temperature is below freezing and the other exceeds boiling point.
- Write a C program that takes two temperatures and returns true if the difference between them exceeds 150 degrees.
- Write a C program that returns true if one of two temperature readings is negative and the other is positive with an absolute value greater than 50.
C Programming Code Editor:
Previous: Write a C program to check whether a given positive number is a multiple of 3 or a multiple of 7.
Next: Write a C program to check two given integers whether either of them is in the range 100..200 inclusive.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.