C Exercises: Check two given integers whether either of them is in the range 100..200 inclusive
7. Range Check 100 to 200
Write a C program to check two given integers whether either of them is in the range 100..200 inclusive.
C Code:
#include <stdio.h> // Include standard input/output library
#include <stdlib.h> // Include standard library for absolute value function
int test(int x, int y); // Declare the function 'test' with two integer parameters
int main(void)
{
// Call the function 'test' with arguments 100 and 199, and print the result
printf("%d", test(100, 199));
// Print a newline for formatting
printf("\n");
// Call the function 'test' with arguments 250 and 300, and print the result
printf("%d", test(250, 300));
// Print a newline for formatting
printf("\n");
// Call the function 'test' with arguments 105 and 190, and print the result
printf("%d", test(105, 190));
}
// Function definition for 'test'
int test(int x, int y)
{
// Check if either of the following conditions are true:
// 1. x is between 100 and 200 (inclusive)
// 2. y is between 100 and 200 (inclusive)
// Return 1 (true) if either condition is true, otherwise return 0 (false).
return (x >= 100 && x <= 200) || (y >= 100 && y <= 200);
}
Sample Output:
1 0 1
Explanation:
int test(int x, int y) { return (x >= 100 && x <= 200) || (y >= 100 && y <= 200); }
This function takes two integer inputs ‘x’ and ‘y’ and returns 1 if either of the inputs is between 100 and 200 (inclusive), and returns 0 otherwise.
Time complexity and space complexity:
Time complexity: O(1) - The function has a constant time complexity, as the same number of operations are performed regardless of the input values.
Space complexity: O(1) - The function uses a constant amount of memory, as the inputs and the return value are the only variables used.
Pictorial Presentation:
Flowchart:
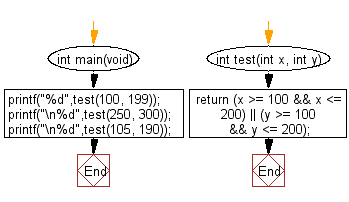
For more Practice: Solve these Related Problems:
- Write a C program to check if at least one of two integers lies within the range 150 to 250.
- Write a C program that verifies if one integer is between 50 and 150 or if both are outside this range.
- Write a C program to determine if either of two numbers falls within the range 100 to 300 inclusive.
- Write a C program to check if both integers fall within the range 90 to 210 and return true if at least one does.
C Programming Code Editor:
Previous: Write a C program to check if one given temperatures is less than 0 and the other is greater than 100.
Next: Write a C program to check whether three given integer values are in the range 20..50 inclusive. Return true if 1 or more of them are in the said range otherwise return false.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.