C Exercises: Check whether three given integer values are in the range 20..50 inclusive
C-programming basic algorithm: Exercise-8 with Solution
Write a C program that checks if three given integers are in the range of 20 to 50 (inclusive) and returns true if at least one of them is within the range. If none of the integers are within the range, the program returns false.
C Code:
#include <stdio.h> // Include standard input/output library
#include <stdlib.h> // Include standard library for additional functions
// Declare the function 'test' with three integer parameters
int test(int x, int y, int z);
int main(void)
{
// Call the function 'test' with arguments 11, 20, and 12, and print the result
printf("%d", test(11, 20, 12));
// Print a newline for formatting
printf("\n");
// Call the function 'test' with arguments 30, 30, and 17, and print the result
printf("%d", test(30, 30, 17));
// Print a newline for formatting
printf("\n");
// Call the function 'test' with arguments 25, 35, and 50, and print the result
printf("%d", test(25, 35, 50));
// Print a newline for formatting
printf("\n");
// Call the function 'test' with arguments 15, 12, and 8, and print the result
printf("%d", test(15, 12, 8));
}
// Function definition for 'test'
int test(int x, int y, int z)
{
// Check if either of the following conditions are true for any of the variables (x, y, or z):
// 1. The variable is between 20 and 50 (inclusive)
// Return 1 (true) if any condition is true, otherwise return 0 (false).
return (x >= 20 && x <= 50) || (y >= 20 && y <= 50) || (z >= 20 && z <= 50);
}
Sample Output:
1 1 1 0
Explanation:
int test(int x, int y, int z) { return (x >= 20 && x <= 50) || (y >= 20 && y <= 50) || (z >= 20 && z <= 50); }
The above function test takes three integer parameters ‘x’, ‘y’, and ‘z’. It checks if at least one of the three integers is in the range of 20 to 50 (inclusive).
If any of the parameters ‘x’, ‘y’, or ‘z’ is greater than or equal to 20 and less than or equal to 50, the function returns 1 (true), indicating that at least one of the numbers is within the specified range. Otherwise, it returns 0 (false), indicating that none of the numbers are within the specified range.
Time complexity and space complexity:
Time complexity: The function performs three comparisons and three logical OR operations, all of which are constant time operations. Therefore, the time complexity of the function is O(1).
Space complexity: The function uses a constant amount of space to store the input parameters and the return value, so the space complexity is also O(1).
Pictorial Presentation:
Flowchart:
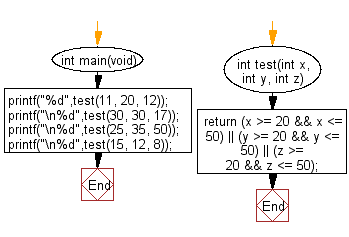
C Programming Code Editor:
Previous: Write a C program to check two given integers whether either of them is in the range 100..200 inclusive.
Next: Write a C program to check whether two given integer values are in the range 20..50 inclusive. Return true if 1 or other is in the said range otherwise false.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/c-programming-exercises/basic-algo/c-programming-basic-algorithm-exercises-8.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics