C Exercises: Display the integer equivalents of letters
Display integer equivalents for all letters (a–z, A–Z)
Write a C program to display the integer equivalents of letters (a-z, A-Z).
Sample Solution:
C Code:
#include<stdio.h>
int main()
{
// Define a string containing lowercase and uppercase letters
char* letters = "abcdefghijklmnopqrstuvwxyz ABCDEFGHIJKLMNOPQRSTUVWXYZ";
int n;
// Print header
printf("List of integer equivalents of letters (a-z, A-Z).\n");
printf("==================================================\n");
// Loop through each character and print its integer equivalent
for(n=0; n<53; n++) {
printf("%d\t", letters[n]);
// Add a newline every 6 characters for better formatting
if((n+1) % 6 == 0)
printf("\n");
}
return 0;
}
Sample Output:
List of integer equivalents of letters (a-z, A-Z). ================================================== 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 32 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90
Flowchart:
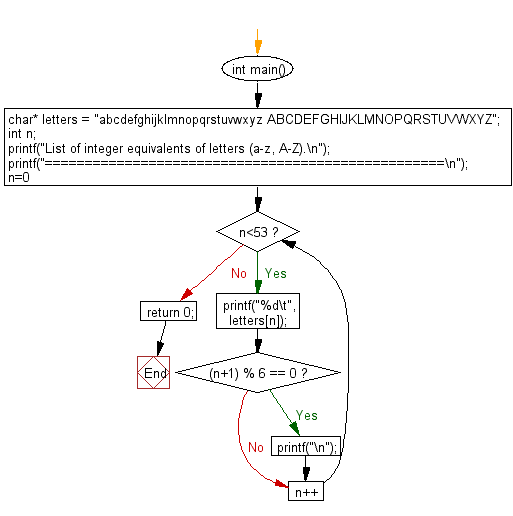
For more Practice: Solve these Related Problems:
- Write a C program to display the ASCII values of letters a–z and A–Z in a tabular format.
- Write a C program to print the ASCII values of alphabets in ascending order with corresponding characters.
- Write a C program to generate a table of letters with ASCII codes and highlight vowels in a different color.
- Write a C program to output the ASCII values of uppercase and lowercase letters sorted by numeric value.
C programming Code Editor:
Previous:Write a C program that reads in two integers and check whether the first integer is a multiple of the second integer.
Next: Write a C program that accepts one seven-digit number and separates the number into its individual digits, and prints the digits separated from one another by two spaces each.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.