C Exercises: Accepts one seven-digit number and separates the number into its individual digits, and prints the digits separated from one another by two spaces each
Separate the digits of a 7-digit number with spaces
Write a C program that accepts a seven-digit number, separates the number into its individual digits, and prints the digits separated from one another by two spaces each.
Sample Input: 2345678
Sample Solution:
C Code:
#include<stdio.h>
int main()
{
int n;
// Prompt for input
printf( "Input a seven digit number: " );
// Read the input
scanf("%d", &n);
// Output header
printf( "\nOutput: " );
// Extract and print each digit
printf("%d ", (n/1000000));
n = n - ((n/1000000)*1000000);
printf("%d ", (n/100000));
n = n - ((n/100000)*100000);
printf("%d ", (n/10000));
n = n - ((n/10000)*10000);
printf("%d ", (n/1000));
n = n - ((n/1000)*1000);
printf("%d ", (n/100));
n = n - ((n/100)*100);
printf("%d ", (n/10));
n = n - ((n/10)*10);
printf("%d\n", (n%10));
return 0;
}
Sample Output:
Input a seven digit number: Output: 2 3 4 5 6 7 8
Pictorial Presentation:
Flowchart:
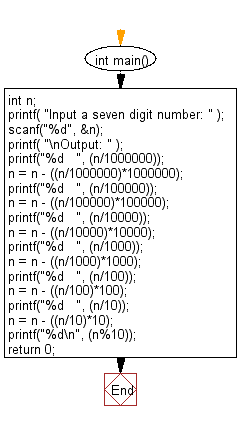
For more Practice: Solve these Related Problems:
- Write a C program to separate and display the digits of an integer using only arithmetic operators.
- Write a C program to print each digit of a 7-digit number on a new line using recursion.
- Write a C program to separate the digits of a number and display them in reverse order with spaces.
- Write a C program to extract and display each digit of a 7-digit number, grouping even and odd digits separately.
C programming Code Editor:
Previous:Write a C program to display the integer equivalents of letters (a-z, A-Z).
Next: Write a C program to calculate and prints the squares and cubes of the numbers from 0 to 20 and uses tabs to display them in a table of values.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.