C Exercises: Largest or smallest element in an array
8. Min/Max Element Callback Variants
Write a C program to find the largest or smallest element in an array using a callback function to compare the elements.
Sample Solution:
C Code:
#include <stdio.h>
int find_max_or_min(int arr[], int size, int( * compare)(int, int)) {
int i, result;
result = arr[0];
for (i = 1; i < size; i++) {
if (compare(result, arr[i]) > 0) {
result = arr[i];
}
}
return result;
}
int compare_min(int a, int b) {
return a - b;
}
int compare_max(int a, int b) {
return b - a;
}
int main() {
int arr[] = {
7,
0,
4,
2,
9,
5,
1
};
int size = sizeof(arr) / sizeof(int);
printf("Original array elements: ");
for (size_t i = 0; i < size; i++) {
printf("%d ", arr[i]);
}
int min = find_max_or_min(arr, size, compare_min);
int max = find_max_or_min(arr, size, compare_max);
printf("\n\nThe minimum element is %d\n", min);
printf("The maximum element is %d\n", max);
return 0;
}
Sample Output:
Original array elements: 7 0 4 2 9 5 1 The minimum element is 0 The maximum element is 9
Explanation:
In the above program, the find_max_or_min() function takes three arguments an array, its size, and a comparison function. It then iterates through the array, comparing each element to the current result using the provided comparison function to determine whether the new element is larger or smaller.
Two comparison functions, compare_min() and compare_max(), are defined to determine whether the result should be the smallest or largest element in the array, respectively.
In the main() function, find_max_or_min() function is called twice, once with compare_min() function to find the smallest element, and once with compare_max() function to find the largest element. The printf() function finally prints the results.
Here the callback function is used to modify the behavior of a function without changing its core logic.
Flowchart:
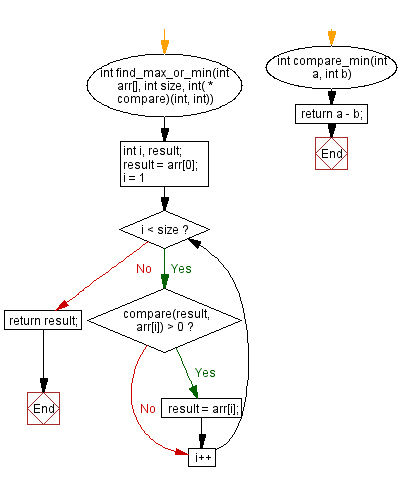
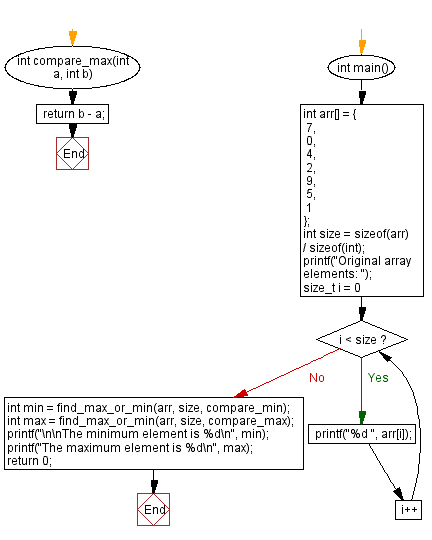
For more Practice: Solve these Related Problems:
- Write a C program to find the second largest element in an array using a callback function.
- Write a C program to determine the element with the smallest absolute value in an array using a callback comparator.
- Write a C program to find both the maximum and minimum elements simultaneously using a single callback function.
- Write a C program to identify the most frequently occurring element in an array using a callback function.
C Programming Code Editor:
Previous: Remove all whitespace from a string.
Next: Shuffle the elements of an array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.