C Exercises: Shuffle the elements of an array
9. Array Shuffle Callback Variants
Write a C program to shuffle the elements of an array using a callback function to generate random numbers for swapping.
Sample Solution:
C Code:
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
void shuffle(int * array, size_t n, int( * rand_func)(void)) {
for (int i = n - 1; i > 0; i--) {
int j = rand_func() % (i + 1);
int tmp = array[i];
array[i] = array[j];
array[j] = tmp;
}
}
int main() {
int arr[] = {
1,
2,
3,
4,
5
};
size_t n = sizeof(arr) / sizeof(int);
printf("Original array elements: ");
for (size_t i = 0; i < n; i++) {
printf("%d ", arr[i]);
}
// Seed the random number generator
srand(time(NULL));
// Shuffle the array using rand() as the random number generator
shuffle(arr, n, rand);
// Print the shuffled array
printf("\nShuffled array elements: ");
for (int i = 0; i < n; i++) {
printf("%d ", arr[i]);
}
printf("\n");
return 0;
}
Sample Output:
Original array elements: 1 2 3 4 5 Shuffled array elements: 3 5 2 1 4
Note: The results may vary due to srand() function is used to seed the random number.
Explanation:
In the above program, the shuffle() function takes three arguments an array of integers, the number of elements in the array, and a callback function that generates a random integer. It then shuffles the elements of the array by iterating over the array backwards and swapping each element with a randomly chosen element from the range 0 to the current index.
In the main() function, an array of integers is created and its elements are shuffled using the shuffle() function. The rand() function is used as the callback function for generating random numbers.
Flowchart:
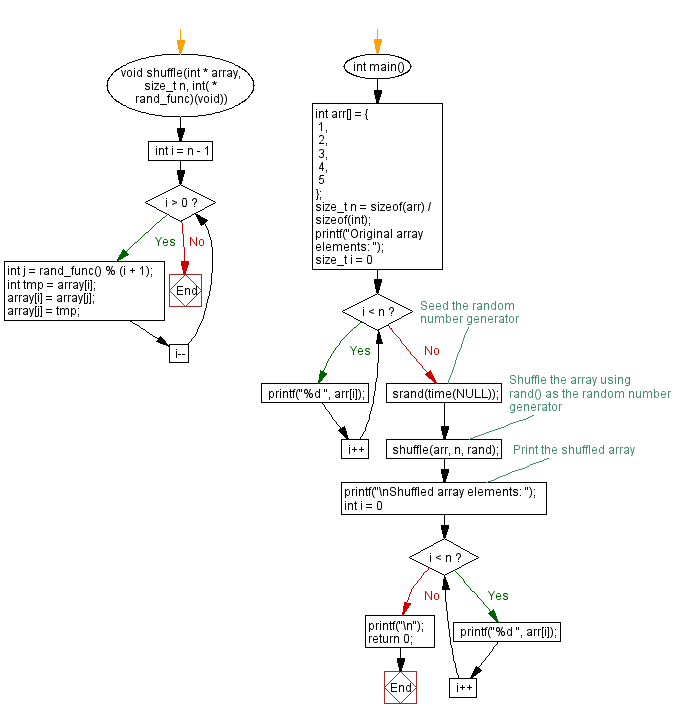
For more Practice: Solve these Related Problems:
- Write a C program to implement the Fisher-Yates shuffle algorithm using a callback function for random number generation.
- Write a C program to shuffle an array and then restore the original order using inverse operations via callbacks.
- Write a C program to shuffle an array and print the new indices of elements using a callback for swapping.
- Write a C program to implement a custom shuffling routine using a callback function that accepts a user-defined seed.
C Programming Code Editor:
Previous: Largest or smallest element in an array.
Next: Quick sort.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.