C Program: Calculate average of user input numbers
7. Average of User Input Numbers
Write a C program that calculates the average of a set of numbers input by the user. The user should be able to input as many numbers as desired, and the program should continue until the user decides to stop.
Sample Solution:
C Code:
#include <stdio.h>
int main() {
int count = 0; // Variable to store the number of entered values
double sum = 0.0; // Variable to store the sum of entered values
double number; // Variable to store the user input
// Prompt the user to enter numbers and calculate the average
printf("Input numbers to calculate the average (enter a non-numeric value to stop):\n");
while (1) {
// Read the user input
printf("Input a number: ");
if (scanf("%lf", &number) != 1) {
// If the input is not a valid number, break out of the loop
break;
}
// Add the entered number to the sum
sum += number;
// Increment the count of entered values
count++;
}
// Check if any numbers were entered
if (count > 0) {
// Calculate and print the average
double average = sum / count;
printf("Average of input numbers: %.2lf\n", average);
} else {
// If no numbers were entered, print a message
printf("No numbers were entered.\n");
}
return 0; // Indicate successful program execution
}
Sample Output:
Input numbers to calculate the average (enter a non-numeric value to stop): Input a number: 3 Input a number: 1 Input a number: 12 Input a number: -12 Input a number: 6 Input a number: a Average of input numbers: 2.00
Explanation:
Here are key parts of the above code step by step:
- int count = 0;: Initializes a variable to store the number of entered values.
- double sum = 0.0;: Initializes a variable to store the sum of entered values.
- double number;: Declares a variable to store user input.
- while (1) { ... }: Creates an infinite loop.
- printf("Enter numbers to calculate the average (enter a non-numeric value to stop):\n");: Displays a message to the user.
- if (scanf("%lf", &number) != 1) { ... }: Reads user input and checks if it's a valid number. If not, breaks out of the loop.
- sum += number;: Adds the entered number to the sum.
- count++;: Increments the count of entered values.
- if (count > 0) { ... }: Checks if any numbers were entered.
- double average = sum / count;: Calculates the average.
- printf("Average of entered numbers: %.2lf\n", average);: Prints the calculated average.
- else { printf("No numbers were entered.\n"); }: If no numbers were entered, prints a message.
- return 0;: Indicates successful program execution.
Flowchart:
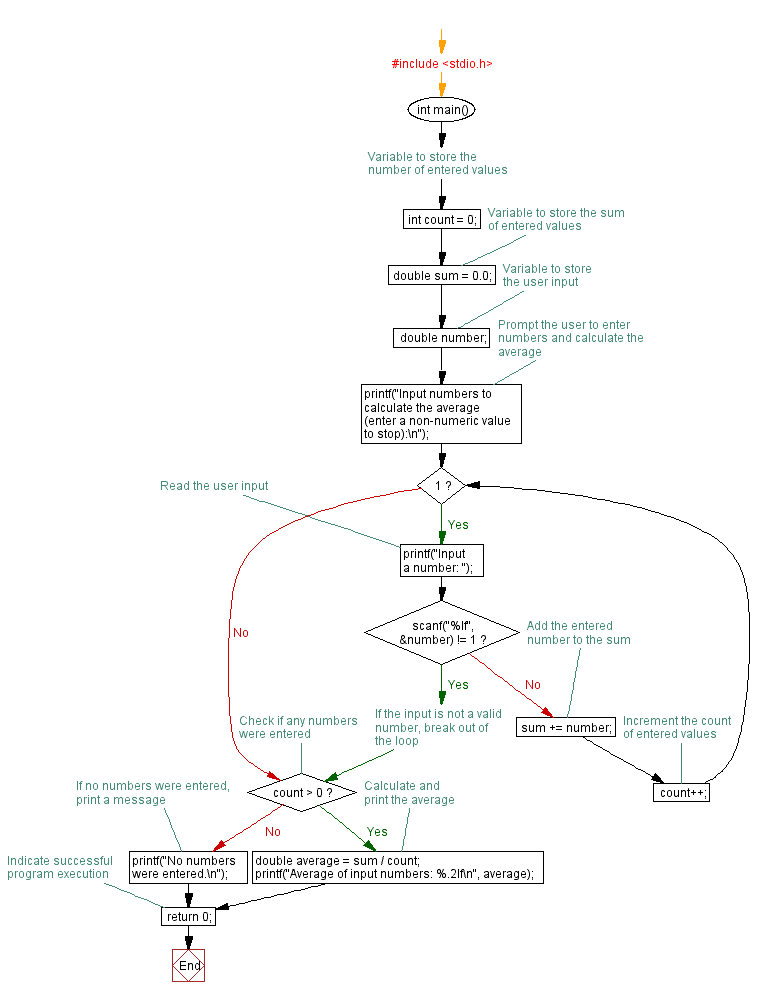
For more Practice: Solve these Related Problems:
- Write a C program to calculate the average of numbers entered by the user but exclude the highest and lowest values.
- Write a C program to calculate the average of numbers entered by the user and round the result to the nearest integer.
- Write a C program to accept numbers until a non-numeric input is entered and compute the average.
- Write a C program to accept numbers until the sum exceeds 500 and then calculate the average.
C Programming Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Calculate Sum of squares of digits with Do-While Loop.
Next: Implement a stack using a singly linked list.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.