C Program: Password authentication with Do-While Loop
C Do-While Loop: Exercise-8 with Solution
Write a C program that prompts the user to enter a password. Use a do-while loop to keep asking for the password until the correct one is entered.
Sample Solution:
C Code:
#include <stdio.h>
#include <string.h>
int main() {
char correctPassword[] = "pass$123"; // Set the correct password
char enteredPassword[20]; // Variable to store the user-entered password
// Use a do-while loop to repeatedly ask for the password
do {
// Prompt the user to enter the password
printf("Input the password: ");
scanf("%s", enteredPassword);
// Check if the entered password is correct
if (strcmp(enteredPassword, correctPassword) == 0) {
printf("Correct password! Access granted.\n");
break; // Exit the loop if the password is correct
} else {
printf("Incorrect password. Try again.\n");
}
} while (1); // Continue the loop indefinitely until the correct password is entered
return 0; // Indicate successful program execution
}
Sample Output:
Input the password: pass123$ Incorrect password. Try again. Input the password: pass123# Incorrect password. Try again. Input the password: 123pass$ Incorrect password. Try again. Input the password: pass123$ Incorrect password. Try again. Input the password: pass$123 Correct password! Access granted.
Explanation:
Here are key parts of the above code step by step:
- char correctPassword[] = "pass$123";: Sets the correct password as a string.
- char enteredPassword[20];: Declares an array to store the user-entered password.
- do { ... } while (1);: Creates a do-while loop that continues indefinitely.
- printf("Enter the password: ");: Prompts the user to enter the password.
- scanf("%s", enteredPassword);: Reads the user's input into the 'enteredPassword' array.
- if (strcmp(enteredPassword, correctPassword) == 0) { ... }: Compares the entered password with the correct password using strcmp.
- printf("Correct password! Access granted.\n");: Displays a success message if the password is correct.
- break;: Exits the loop if the correct password is entered.
- else { printf("Incorrect password. Try again.\n"); }: Displays an error message if the password is incorrect.
- } while (1);: Continues the loop until the correct password is entered.
- return 0;: Indicates successful program execution.
Flowchart:
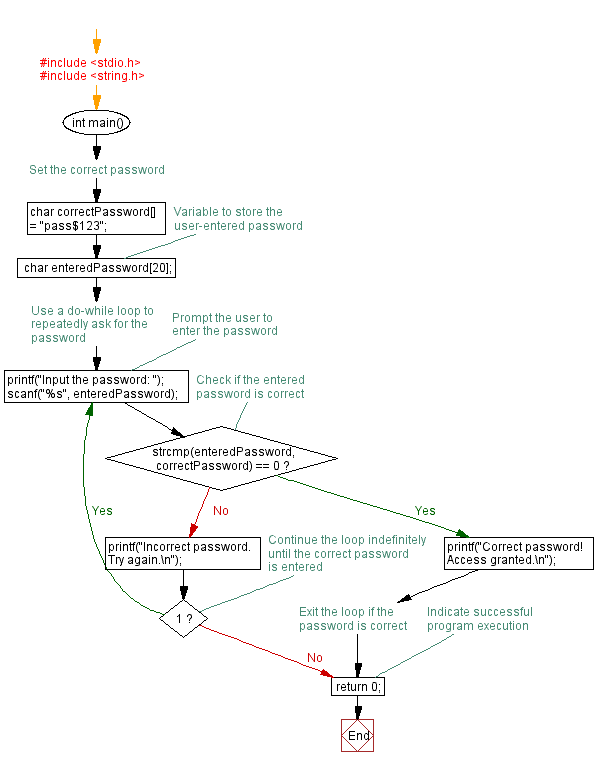
C Programming Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Calculate average of user input numbers.
Next: Calculate sum of Prime numbers with Do-While Loop.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics