C Program: Calculate sum of Prime numbers with Do-While Loop
C Do-While Loop: Exercise-9 with Solution
Write a C program that calculates and prints the sum of prime numbers up to a specified limit (e.g., 50) using a do-while loop.
Sample Solution:
C Code:
#include <stdio.h>
int isPrime(int num) {
if (num < 2) {
return 0; // Not a prime number
}
for (int i = 2; i * i <= num; i++) {
if (num % i == 0) {
return 0; // Not a prime number
}
}
return 1; // Prime number
}
int main() {
int limit;
int num = 2; // Starting from the first prime number
int sum = 0;
// Prompt the user to enter a limit
printf("Input the limit for prime numbers: ");
scanf("%d", &limit);
// Calculate and print the sum of prime numbers up to the specified limit
do {
if (isPrime(num)) {
sum += num; // Add prime numbers to the sum
}
num++; // Move to the next number
} while (num <= limit);
// Print the sum of prime numbers
printf("Sum of prime numbers up to %d: %d\n", limit, sum);
return 0; // Indicate successful program execution
}
Sample Output:
Input the limit for prime numbers: 50 Sum of prime numbers up to 50: 328
Input the limit for prime numbers: 7 Sum of prime numbers up to 7: 17
Explanation:
Here are key parts of the above code step by step:
- int isPrime(int num): Function to check if a number is prime. Returns 1 for prime numbers, 0 otherwise.
- int limit;: Variable to store the user-specified limit.
- int num = 2;: Initializes the variable to start checking prime numbers from 2.
- int sum = 0;: Initializes the variable to store the sum of prime numbers.
- printf("Enter the limit for prime numbers: ");: Prompts the user to enter the limit.
- scanf("%d", &limit);: Reads the user-specified limit.
- do { ... } while (num <= limit);: Do-while loop to calculate the sum of prime numbers up to the specified limit.
- if (isPrime(num)) { sum += num; }: If the current number is prime, add it to the sum.
- num++;: Move to the next number.
- } while (num <= limit);: Continue the loop until the specified limit is reached.
- printf("Sum of prime numbers up to %d: %d\n", limit, sum);: Print the sum of prime numbers.
- return 0;: Indicates successful program execution.
Flowchart:
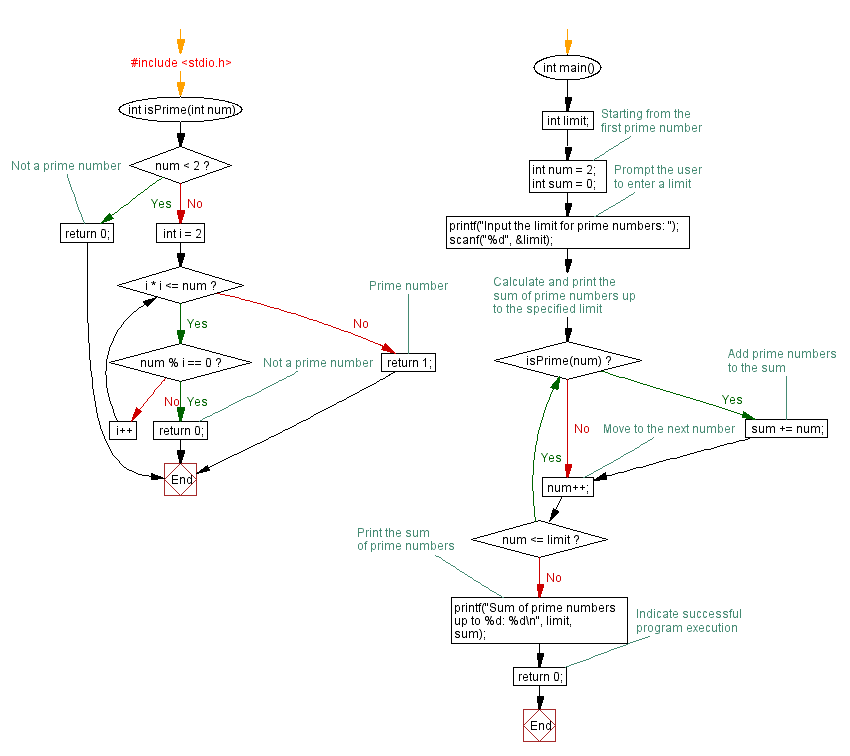
C Programming Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Password authentication with Do-While Loop.
Next: Counting digits in an integer using Do-While Loop.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/c-programming-exercises/do-while-loop/c-do-while-loop-exercise-9.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics