C Program: Counting digits in an integer using Do-While Loop
C Do-While Loop: Exercise-10 with Solution
Write a C program that implements a program to count the number of digits in a given integer using a do-while loop.
Sample Solution:
C Code:
#include <stdio.h>
int main() {
int num; // Declare a variable to store the input number
int digitCount = 0; // Initialize the count of digits
// Prompt the user to enter an integer
printf("Input an integer: ");
scanf("%d", &num);
// Take the absolute value of the entered number
num = (num < 0) ? -num : num;
// Handle the special case when the number is 0
if (num == 0) {
digitCount = 1;
} else {
// Count the number of digits using a do-while loop
do {
digitCount++;
num /= 10;
} while (num != 0);
}
// Print the count of digits
printf("Number of digits: %d\n", digitCount);
return 0; // Indicate successful program execution
}
Sample Output:
Input an integer: 12345 Number of digits: 5
Input an integer: -789 Number of digits: 3
Input an integer: 000 Number of digits: 1
Explanation:
Here are key parts of the above code step by step:
- int num;: Declares a variable to store the input number.
- int digitCount = 0;: Initializes a variable to store the count of digits.
- printf("Enter an integer: ");: Prompts the user to input an integer.
- scanf("%d", &num);: Reads the entered integer from the user.
- (num < 0) ? -num : num;: Takes the absolute value of the entered number.
- if (num == 0) { digitCount = 1; }: Handles the special case when the entered number is 0.
- do { ... } while (num != 0);: Uses a do-while loop to count the number of digits.
- digitCount++;: Increments the digit count for each digit encountered.
- num /= 10;: Removes the last digit from the number.
- printf("Number of digits: %d\n", digitCount);: Prints the count of digits.
- return 0;: Indicates successful program execution.
Flowchart:
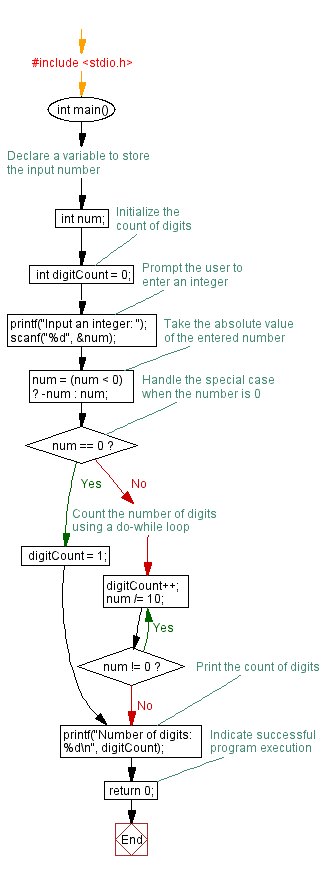
C Programming Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Calculate sum of Prime numbers with Do-While Loop.
Next: Calculating compound interest with User input Loop.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics