C Program: Calculating compound interest with User input Loop
C Do-While Loop: Exercise-11 with Solution
Write a C program that calculates the compound interest for a given principal amount, interest rate, and time period. Use a do-while loop to allow the user to input values multiple times.
Sample Solution:
C Code:
#include <stdio.h>
#include <math.h>
int main() {
double principal, rate, time, compoundInterest;
int flag;
do {
// Prompt the user to enter principal amount, interest rate, and time period
printf("Input principal amount: ");
scanf("%lf", &principal);
printf("Input annual interest rate (as a percentage): ");
scanf("%lf", &rate);
printf("Input time period (in years): ");
scanf("%lf", &time);
// Check if the entered values are valid
if (principal <= 0 || rate < 0 || time <= 0) {
printf("Please input valid positive values for principal, interest rate, and time.\n");
continue; // Restart the loop for new input
}
// Convert interest rate from percentage to decimal
rate /= 100;
// Calculate compound interest
compoundInterest = principal * pow(1 + rate, time) - principal;
// Print the calculated compound interest
printf("Compound Interest: %.2lf\n", compoundInterest);
// Ask the user if they want to calculate again
printf("Want to calculate compound interest again? (1 for Yes, 0 for No): ");
scanf("%d", &flag);
} while (flag == 1);
return 0; // Indicate successful program execution
}
Sample Output:
Input principal amount: 10000 Input annual interest rate (as a percentage): 4 Input time period (in years): 5 Compound Interest: 2166.53 Want to calculate compound interest again? (1 for Yes, 0 for No): 1 Input principal amount: 5000 Input annual interest rate (as a percentage): 3.9 Input time period (in years): 8 Compound Interest: 1790.38 Want to calculate compound interest again? (1 for Yes, 0 for No): 0
Explanation:
Here are key parts of the above code step by step:
- double principal, rate, time, compoundInterest; int flag: Declares variables to store principal amount, interest rate, time period, and compound interest. Using 'flag' ask the user if they want to calculate again.
- do { ... } while (time == 1);: Uses a do-while loop to allow the user to input values multiple times.
- principal amount: ");, scanf("%lf", &principal);, etc.: Prompts the user to input principal amount, interest rate, and time period.
- if (principal <= 0 || rate < 0 || time <= 0) { ... }: Checks if the input values are valid. If not, prompts the user to enter valid positive values.
- rate /= 100;: Converts the interest rate from percentage to decimal.
- compoundInterest = principal * pow(1 + rate, time) - principal;: Calculates compound interest using the compound interest formula.
- printf("Compound Interest: %.2lf\n", compoundInterest);: Prints the calculated compound interest.
- printf("Do you want to calculate compound interest again? (Enter 1 for Yes, 0 for No): ");, scanf("%d", &time);: Asks the user if they want to calculate again. If the user enters 1, the loop continues; otherwise, it exits.
- return 0;: Indicates successful program execution.
Flowchart:
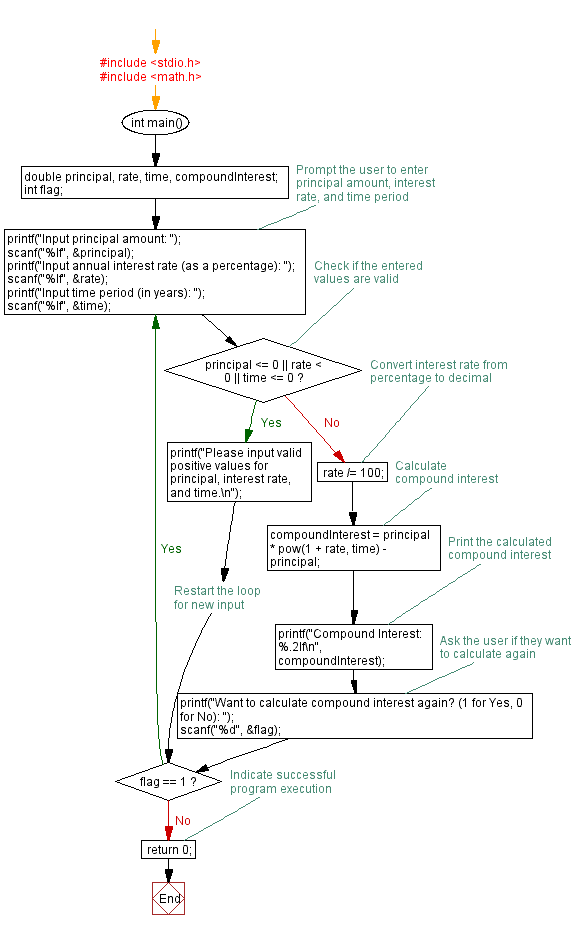
C Programming Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Counting digits in an integer using Do-While Loop.
Next: Reversing a number using a Do-While loop.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics