C Program: Reversing a number using a Do-While loop
12. Reverse a Number
Write a C program to reverse a given number using a do-while loop.
Sample Solution:
C Code:
#include <stdio.h>
int main() {
int num, reversedNum = 0, remainder;
// Prompt the user to enter a number
printf("Input a number: ");
scanf("%d", &num);
// Store the original number in a separate variable
int originalNum = num;
// Reverse the number using a do-while loop
do {
// Extract the last digit
remainder = num % 10;
// Build the reversed number by appending the extracted digit
reversedNum = reversedNum * 10 + remainder;
// Remove the last digit from the original number
num = num / 10;
} while (num != 0);
// Print the original and reversed numbers
printf("Original Number: %d\n", originalNum);
printf("Reversed Number: %d\n", reversedNum);
return 0; // Indicate successful program execution
}
Sample Output:
Input a number: 235235 Original Number: 235235 Reversed Number: 532532
Input a number: -1234 Original Number: -1234 Reversed Number: -4321
Explanation:
Here are key parts of the above code step by step:
- int num, reversedNum = 0, remainder;: Declares variables to store the original number (num), reversed number ('reversedNum'), and remainder.
- printf("Input a number: ");, scanf("%d", &num);: Prompts the user to enter a number and reads it.
- int originalNum = num;: Stores the original number in a separate variable.
- do { ... } while (num != 0);: Uses a do-while loop to reverse the number.
- remainder = num % 10;: Extracts the last digit of the number.
- reversedNum = reversedNum * 10 + remainder;: Builds the reversed number by appending the extracted digit.
- num = num / 10;: Removes the last digit from the original number.
- printf("Original Number: %d\n", originalNum);, printf("Reversed Number: %d\n", reversedNum);: Prints the original and reversed numbers.
- return 0;: Indicates successful program execution.
Flowchart:
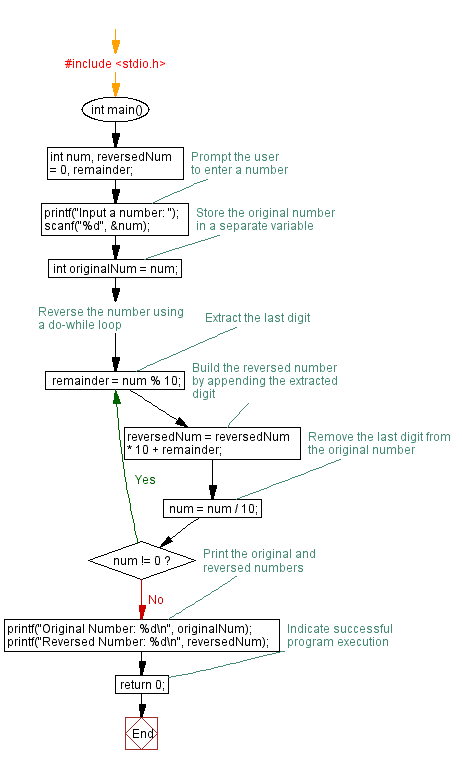
For more Practice: Solve these Related Problems:
- Write a C program to reverse a number without using modulus (%) or division (/) operators.
- Write a C program to reverse a number and check if the reversed number is a prime number.
- Write a C program to reverse a number and replace all occurrences of digit '3' with '8' in the reversed number.
- Write a C program to reverse a number and then check if the original number and reversed number form a palindrome pair.
C Programming Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Calculating compound interest with User input Loop.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.