C Exercises: Display n natural numbers and their sum
3. Display n Terms of Natural Numbers and Their Sum
Write a program in C to display n terms of natural numbers and their sum.
This C program displays the first 'n' natural numbers and calculates their sum. It prompts the user to input the value of 'n', then uses a "for" loop to iterate through the numbers, printing each one and accumulating the total sum, which is displayed at the end. This task reinforces the use of loops, user input, and basic arithmetic in C programming.
Visual Presentation:
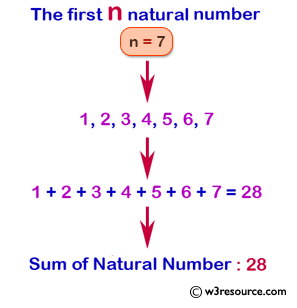
Sample Solution:
C Code:
#include <stdio.h> // Include the standard input/output header file.
void main() {
int i, n, sum = 0; // Declare variables 'i' for loop counter, 'n' for the number of terms, and 'sum' to store the sum.
printf("Input Value of terms : "); // Print a message to prompt user input.
scanf("%d", &n); // Read the value of 'n' from the user.
printf("\nThe first %d natural numbers are:\n", n); // Print a message to indicate the output.
for (i = 1; i <= n; i++) { // Start a for loop to iterate from 1 to 'n'.
printf("%d ", i); // Print the current value of 'i'.
sum += i; // Add the current value of 'i' to the sum.
}
printf("\nThe Sum of natural numbers upto %d terms : %d \n", n, sum); // Print the sum of natural numbers.
}
Output:
Input Value of terms : 7 The first 7 natural number is : 1 2 3 4 5 6 7 The Sum of Natural Number upto 7 terms : 28
Explanation:
for (i = 1; i <= n; i++) { printf("%d ", i); sum += i; }
In the above for loop, the variable i is initialized to 1, and the loop will continue as long as i is less than or equal to the value of variable 'n'. In each iteration of the loop, the printf() function will print the value of i to the console, followed by a space character. Additionally, the value of i will be added to the variable 'sum' in each iteration of the loop.
Finally, the loop will increment the value of i by 1, and the process will repeat until the condition i<=n is no longer true.
Flowchart:
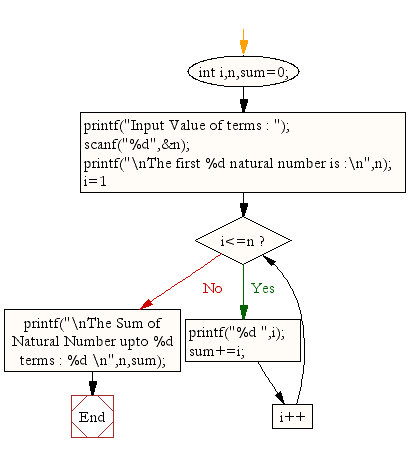
For more Practice: Solve these Related Problems:
- Write a C program to display n terms of natural numbers using recursion and compute their cumulative sum at each step.
- Write a C program that takes n as input and displays the natural numbers in reverse order along with their total sum.
- Write a C program to dynamically allocate memory for n natural numbers, display them, and then calculate the sum using pointer arithmetic.
- Write a C program to display n natural numbers and print the running total after each number is output.
C Programming Code Editor:
Previous: Write a C program to find the sum of first 10 natural numbers.
Next: Write a program in C to read 10 numbers from keyboard and find their sum and average.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.