C Exercises: Read 10 numbers and find their sum and average
4. Sum and Average of 10 Numbers from Keyboard
Write a program in C to read 10 numbers from the keyboard and find their sum and average.
This C program reads 10 numbers from the keyboard, calculates their sum, and computes their average. It uses a "for" loop to collect user input for each number, accumulates the total sum, and then divides the sum by 10 to find the average. This exercise demonstrates the use of loops, arrays, and arithmetic operations in C programming.
Visual Presentation:
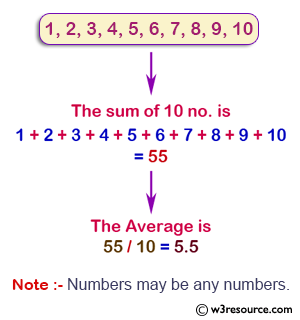
Pseudo code:
initialize sum = 0 initialize ctr = 0 for i = 1 to 10 do read number[i] sum = sum + number[i] ctr = ctr + 1 end for average = sum / ctr print "Sum of the 10 numbers is ", sum print "Average of the 10 numbers is ", average
Sample Solution:
C Code:
#include <stdio.h> // Include the standard input/output header file.
void main() {
int i, n, sum = 0; // Declare variables 'i' for loop counter, 'n' for user input, 'sum' to store the sum.
float avg; // Declare variable 'avg' to store the average.
printf("Input the 10 numbers : \n"); // Print a message to prompt user input.
for (i = 1; i <= 10; i++) { // Start a for loop to iterate 10 times.
printf("Number-%d :", i); // Print a message to indicate which number is being input.
scanf("%d", &n); // Read the value of 'n' from the user.
sum += n; // Add the value of 'n' to the running sum.
}
avg = sum / 10.0; // Calculate the average.
printf("The sum of 10 no is : %d\nThe Average is : %f\n", sum, avg); // Print the sum and average.
}
class="samp-out">Output:
Input the 10 numbers : Number-1 :1 Number-2 :2 Number-3 :3 Number-4 :4 Number-5 :5 Number-6 :6 Number-7 :7 Number-8 :8 Number-9 :9 Number-10 :10 The sum of 10 no is : 55 The Average is : 5.500000
Explanation:
for (i = 1; i <= 10; i++) { printf("Number-%d :", i); scanf("%d", & n); sum += n; }
In the above for loop, the variable i is initialized to 1, and the loop will continue as long as i is less than or equal to 10. In each iteration of the loop, the printf() function will print the string "Number-" followed by the value of i and a colon (:) to the console.
Then, the scanf() function will wait for the user to input an integer value, which will be stored in the variable 'n'. The value of 'n' will then be added to the variable 'sum' in each iteration of the loop.
Finally, the loop will increment the value of i by 1, and the process will repeat until the condition i<=10 is no longer true.
Flowchart
>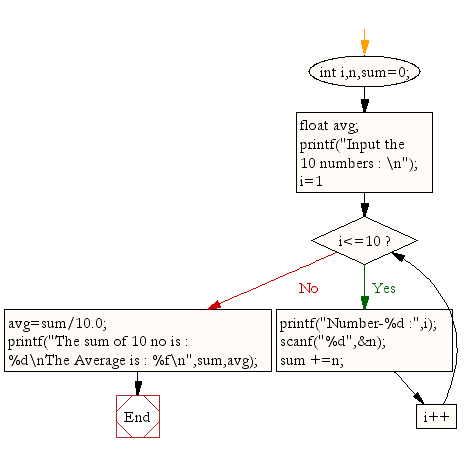
For more Practice: Solve these Related Problems:
- Write a C program to read 10 floating-point numbers and calculate their sum and average using pointers.
- Write a C program to read 10 numbers without using arrays and compute the sum and average by storing each in separate variables.
- Write a C program to read 10 numbers, then determine and display the maximum, minimum, sum, and average.
- Write a C program to read 10 numbers and calculate the sum and average, then display the median value as well.
C Programming Code Editor:
Previous: Write a program in C to display n terms of natural number and their sum.
Next: Write a program in C to display the cube of the number upto a given integer.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.