C Exercises: Find cube of the number upto a given integer
C For Loop: Exercise-5 with Solution
Write a program in C to display the cube of the number up to an integer.
This C program calculates and displays the cube of each number up to a given integer. It uses a "for" loop to iterate through each number from 1 to the specified integer, computes the cube by raising the number to the power of three, and then prints the result.
Visual Presentation:
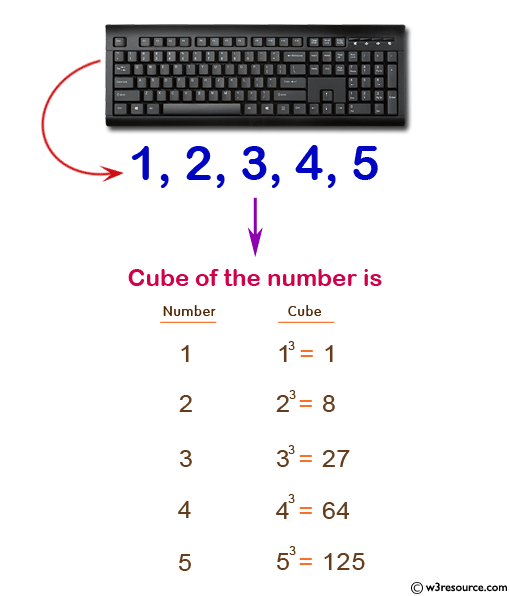
Sample Solution:
C Code:
#include <stdio.h> // Include the standard input/output header file.
void main() {
int i, ctr; // Declare variables 'i' for loop counter and 'ctr' for user input.
printf("Input number of terms : "); // Print a message to prompt user input.
scanf("%d", &ctr); // Read the value of 'ctr' from the user.
for (i = 1; i <= ctr; i++) { // Start a for loop to iterate 'ctr' times.
printf("Number is : %d and cube of the %d is :%d \n", i, i, (i * i * i)); // Print the number, its cube, and message.
}
}
Output:
Input number of terms : 5 Number is : 1 and cube of the 1 is :1 Number is : 2 and cube of the 2 is :8 Number is : 3 and cube of the 3 is :27 Number is : 4 and cube of the 4 is :64 Number is : 5 and cube of the 5 is :125
Explanation:
for (i = 1; i <= ctr; i++) { printf("Number is : %d and cube of the %d is :%d \n", i, i, (i * i * i)); }
In the above for loop, the variable i is initialized to 1, and the loop will continue as long as i is less than or equal to the value of variable 'ctr'. In each iteration of the loop, the printf function will print a formatted string to the console. The string will display the value of i twice and the cube of i.
The first placeholder %d will be replaced by the value of i, the second placeholder %d will also be replaced by the value of i, and the third placeholder %d will be replaced by the cube of i (i.e., i * i * i).
The loop will increment the value of i by 1, and the process will repeat until the condition i<=ctr is no longer true.
Flowchart:
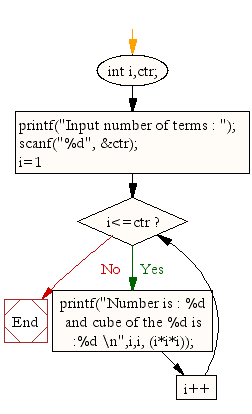
C Programming Code Editor:
Previous: Write a program in C to read 10 numbers from keyboard and find their sum and average.
Next: Write a program in C to display the multiplication table of a given integer.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://www.w3resource.com/c-programming-exercises/for-loop/c-for-loop-exercises-5.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics