C Exercises: Determine the HCF of two numbers
C For Loop: Exercise-43 with Solution
Write a C program to find the HCF (Highest Common Factor) of two numbers.
Visual Presentation:
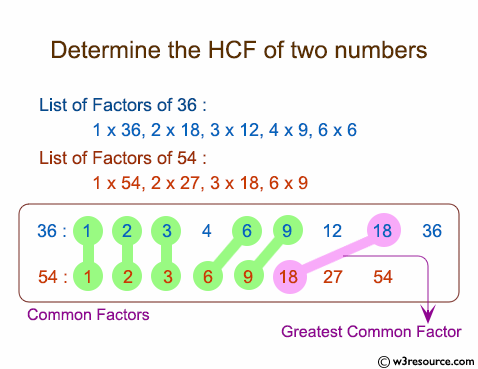
Sample Solution:
C Code:
#include <stdio.h> // Include the standard input/output header file.
void main()
{
int i, n1, n2, j, hcf=1; // Declare variables to store input and results.
printf("\n\n HCF of two numbers:\n "); // Print a message.
printf("----------------------\n"); // Print a separator.
printf("Input 1st number for HCF: "); // Prompt the user for input.
scanf("%d", &n1); // Read the first number from the user.
printf("Input 2nd number for HCF: "); // Prompt the user for input.
scanf("%d", &n2); // Read the second number from the user.
j = (n1 < n2) ? n1 : n2; // Determine the smaller of the two numbers.
// Loop to find the highest common factor (HCF).
for(i = 1; i <= j; i++)
{
if(n1 % i == 0 && n2 % i == 0)
{
hcf = i; // Update the HCF whenever a common factor is found.
}
}
// Print the result.
printf("\nHCF of %d and %d is : %d\n\n", n1, n2, hcf);
}
Sample Output:
HCF of two numbers: ---------------------- Input 1st number for HCF: 24 Input 2nd number for HCF: 28 HCF of 24 and 28 is : 4
Flowchart:
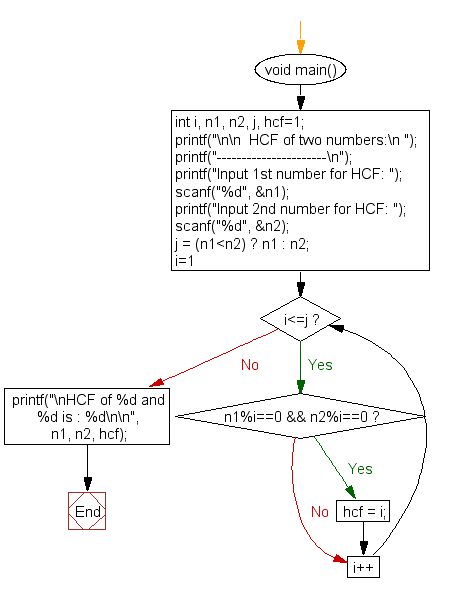
C Programming Code Editor:
Previous: Write a program in C to convert a binary number into a decimal number without using array, function and while loop.
Next: Write a program in C to find LCM of any two numbers using HCF.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics