C Exercises: Convert a binary to a decimal using for loop and without using array
42. Binary to Decimal Without Array, Function, or While Loop
Write a C program to convert a binary number into a decimal number without using array, function and while loop.
The task is to write a C program that converts a given binary number into its decimal representation. The solution should not use arrays, functions, or while loops, focusing instead on using basic control structures like “for” loops and arithmetic operations to achieve the conversion directly within the “main()” function.
Visual Presentation:
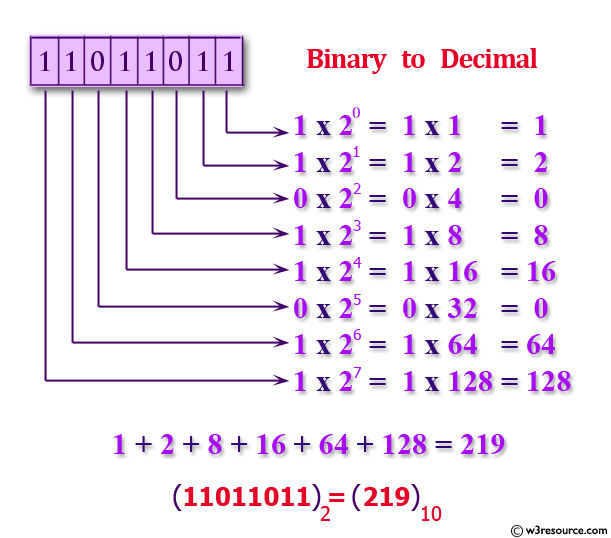
Sample Solution:
C Code:
#include <stdio.h> // Include the standard input/output header file.
void main()
{
int n1, n, p=1; // Declare variables to store input and intermediate results.
int dec=0, i=1, j, d; // Declare variables for decimal and binary conversion.
printf("\n\n Convert Binary to Decimal:\n "); // Print a message.
printf("-------------------------\n"); // Print a separator.
printf("Input a binary number :"); // Prompt the user for input.
scanf("%d", &n); // Read the binary number from the user.
n1 = n; // Store a copy of the original binary number.
// Loop to convert binary to decimal.
for (j = n; j > 0; j = j / 10)
{
d = j % 10; // Get the rightmost digit.
if (i == 1)
p = p * 1;
else
p = p * 2;
dec = dec + (d * p); // Calculate the decimal equivalent.
i++; // Increment the position.
}
// Print the results.
printf("\nThe Binary Number : %d\nThe equivalent Decimal Number : %d \n\n", n1, dec);
}
Output:
Convert Binary to Decimal: ------------------------- Input a binary number :11001 The Binary Number : 11001 The equivalent Decimal Number : 25
Flowchart:
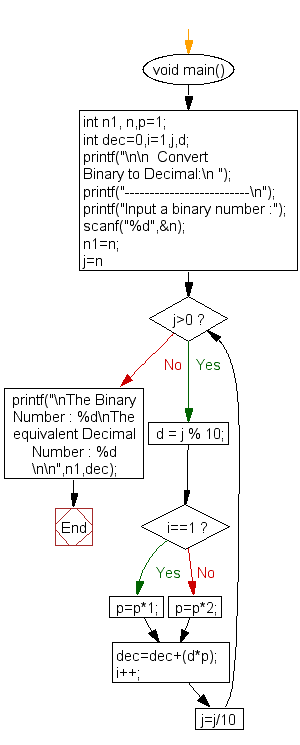
For more Practice: Solve these Related Problems:
- Write a C program to convert a binary literal to decimal using only for loops.
- Write a C program to convert a binary number to decimal using arithmetic operations without arrays.
- Write a C program to convert a binary number to decimal recursively without using a while loop.
- Write a C program to convert a binary number (provided as a literal) to decimal using only switch-case constructs.
C Programming Code Editor:
Previous: Write a program in C to convert a decimal number into binary without using an array.
Next: Write a C program to find HCF (Highest Common Factor) of two numbers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.