C Exercises: Convert a decimal number to binary without using an array
41. Decimal to Binary (Without Array)
Write a program in C to convert a decimal number into binary without using an array.
The task is to write a C program that converts a given decimal number into its binary representation. The solution should not utilize arrays to store intermediate values, focusing instead on using arithmetic and logical operations to generate and display the binary equivalent directly.
Visual Presentation:
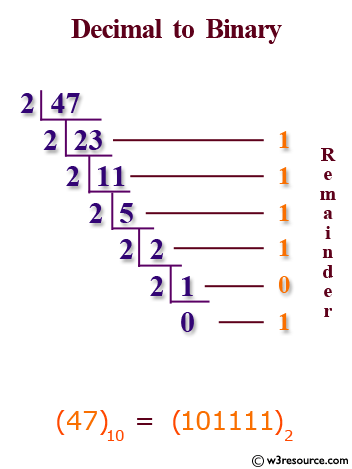
Sample Solution:
C Code:
#include <stdio.h> // Include the standard input/output header file.
#include <stdlib.h> // Include the standard library header file for dynamic memory allocation.
// Function to convert decimal to binary.
char *decimal_to_binary(int dn)
{
int i, j, temp; // Declare variables for iteration and temporary storage.
char *ptr; // Declare a pointer to hold the binary string.
temp = 0; // Initialize temp variable.
ptr = (char*)malloc(32+1); // Allocate memory for a string of maximum 32 bits.
// Loop to convert decimal to binary.
for (i = 31 ; i >= 0 ; i--)
{
j = dn >> i; // Right shift dn by i positions.
if (j & 1) // Check if the rightmost bit is 1.
*(ptr+temp) = 1 + '0'; // Store '1' in the string.
else
*(ptr+temp) = 0 + '0'; // Store '0' in the string.
temp++; // Increment temp.
}
*(ptr+temp) = '\0'; // Add null terminator to the end of the string.
return ptr; // Return the binary string.
}
int main()
{
int dn; // Declare a variable to hold the decimal number.
char *ptr; // Declare a pointer to hold the binary string.
printf("Input a decimal number: ");
scanf("%d", &dn); // Prompt user for input and store it in dn.
ptr = decimal_to_binary(dn); // Call the function to convert decimal to binary.
printf("Binary number equivalent to said decimal number is: %s", ptr); // Print the binary string.
free(ptr); // Free the dynamically allocated memory.
return 0; // Indicate successful program execution.
}
Output:
Input a decimal number: 25 Binary number equivalent to said decimal number is: 0000000000000000000000000001 1001 -------------------------------- Process exited after 3.388 seconds with return value 0 Press any key to continue . . .
Input a decimal number: 105 Binary number equivalent to said decimal number is: 0000000000000000000000000110 1001 -------------------------------- Process exited after 5.013 seconds with return value 0 Press any key to continue . . .
Input a decimal number: 1105 Binary number equivalent to said decimal number is: 0000000000000000000001000101 0001 -------------------------------- Process exited after 17.33 seconds with return value 0 Press any key to continue . . .
Flowchart:
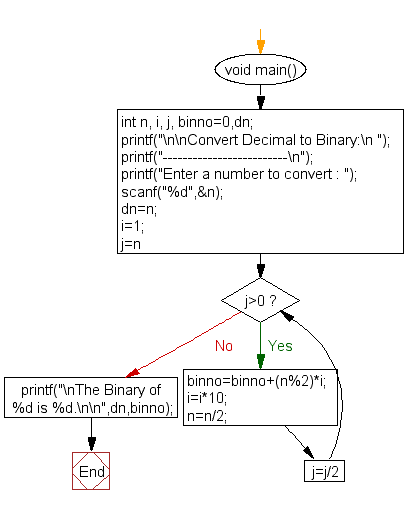
For more Practice: Solve these Related Problems:
- Write a C program to convert a decimal number to binary without using arrays by applying recursion.
- Write a C program to convert a decimal number to binary without arrays and print each binary digit immediately.
- Write a C program to convert a decimal number to binary without arrays and count the number of 1’s in the result.
- Write a C program to convert a decimal number to binary without using arrays and display the output in groups of four digits.
C Programming Code Editor:
Previous: Write a C Program to display the pattern like pyramid using the alphabet.
Next: Write a program in C to convert a binary number into a decimal number without using array, function and while loop.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.