C Exercises: Display the sum of n number of odd natural number
8. Odd Natural Numbers and Their Sum
Write a C program to display the n terms of odd natural numbers and their sum.
like: 1 3 5 7 ... n
This C program displays the first 'n' odd natural numbers and calculates their sum. The user inputs the value of 'n', and the program generates a sequence of odd numbers starting from 1. Using a "for" loop, it sums these odd numbers and displays both the sequence and the total sum.
Visual Presentation:
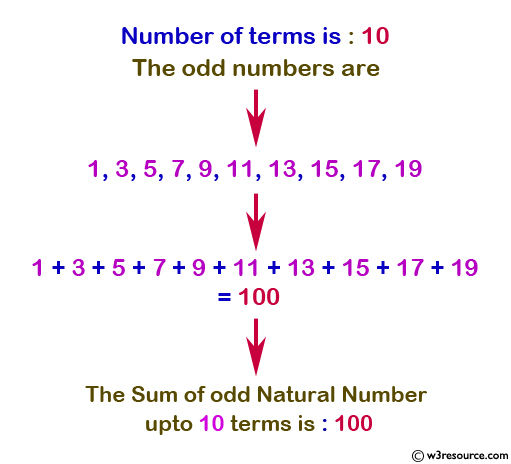
Sample Solution:
C Code:
#include <stdio.h> // Include the standard input/output header file.
void main() {
int i, n, sum = 0; // Declare variables 'i' for loop counter, 'n' for user input, and 'sum' to store the sum.
printf("Input number of terms : "); // Print a message to prompt user input.
scanf("%d", &n); // Read the value of 'n' from the user.
printf("\nThe odd numbers are :"); // Print a message indicating that odd numbers will be displayed.
for (i = 1; i <= n; i++) { // Start a loop to generate odd numbers based on user input.
printf("%d ", 2 * i - 1); // Print the odd number.
sum += 2 * i - 1; // Add the odd number to the running sum.
}
printf("\nThe Sum of odd Natural Number upto %d terms : %d \n", n, sum); // Print the sum of odd numbers.
}
Output:
Input number of terms : 10 The odd numbers are :1 3 5 7 9 11 13 15 17 19 The Sum of odd Natural Number upto 10 terms : 100
Explanation:
for (i = 1; i <= n; i++) { printf("%d ", 2 * i - 1); sum += 2 * i - 1; }
In the said loop, the variable i is initialized to 1, and the loop will continue as long as i is less than or equal to the value of variable 'n'. In each iteration of the loop, the printf function will print the value of (2*i-1) to the console, followed by a space character.
Additionally, the value of (2*i-1) will be added to the variable 'sum' in each iteration of the loop.
The loop will increment the value of i by 1, and the process will repeat until the condition i<=n is no longer true.
Flowchart:
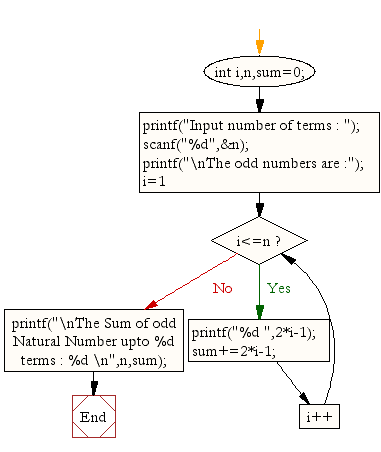
For more Practice: Solve these Related Problems:
- Write a C program to display the first n odd natural numbers and compute their product instead of sum.
- Write a C program to display odd natural numbers up to n and calculate both their sum and average.
- Write a C program to display odd natural numbers in reverse order up to n and then compute the sum.
- Write a C program to generate odd natural numbers recursively and display their cumulative sum at each term.
C Programming Code Editor:
Previous: Write a program in C to display the multiplication table vertically from 1 to n.
Next: Write a program in C to display the pattern like right angle triangle using an asterisk
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.